使用swift语言统计学生的考试成绩:输入n个学生,要求统计每个学生安卓应用开发、IOS应用开发、Web前端程序设计的平均、最高、最低成绩,以及打印出每个学生的各科成绩。
时间: 2023-12-16 10:52:08 浏览: 29
以下是一个简单的实现,假设每个学生只有三门课程:
```
struct Student {
var name: String
var androidScore: Double
var iosScore: Double
var webScore: Double
var averageScore: Double {
return (androidScore + iosScore + webScore) / 3
}
var highestScore: Double {
return max(androidScore, iosScore, webScore)
}
var lowestScore: Double {
return min(androidScore, iosScore, webScore)
}
func printScores() {
print("\(name): Android - \(androidScore), iOS - \(iosScore), Web - \(webScore)")
}
}
func calculateScores() {
print("Enter number of students:")
guard let numberOfStudents = Int(readLine()!) else {
print("Invalid input")
return
}
var students = [Student]()
for i in 1...numberOfStudents {
print("Enter name for student \(i):")
guard let name = readLine() else {
print("Invalid input")
return
}
print("Enter Android score for \(name):")
guard let androidScore = Double(readLine()!) else {
print("Invalid input")
return
}
print("Enter iOS score for \(name):")
guard let iosScore = Double(readLine()!) else {
print("Invalid input")
return
}
print("Enter Web score for \(name):")
guard let webScore = Double(readLine()!) else {
print("Invalid input")
return
}
let student = Student(name: name, androidScore: androidScore, iosScore: iosScore, webScore: webScore)
students.append(student)
}
for student in students {
print("Name: \(student.name)")
print("Average Score: \(student.averageScore)")
print("Highest Score: \(student.highestScore)")
print("Lowest Score: \(student.lowestScore)")
student.printScores()
print()
}
}
calculateScores()
```
这段代码首先要求输入学生数量,然后循环读取每个学生的姓名和成绩,并将每个学生存储在一个 `Student` 结构体中。最后,它循环遍历所有学生,计算并打印每个学生的平均、最高和最低分数,以及打印每个学生的各科成绩。
相关推荐
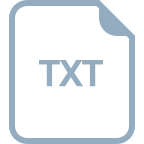














