安卓开发 ExpandableListView 使用示例
时间: 2024-01-24 20:05:29 浏览: 117
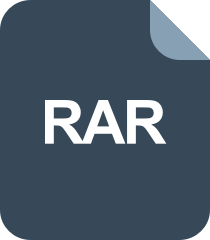
ExpandableListView使用示例

好的,您想了解安卓开发 ExpandableListView 的使用示例,以下是一个简单的示例:
1. 定义适配器类
```
public class ExpandableListAdapter extends BaseExpandableListAdapter {
private Context mContext;
private List<String> mGroupTitles; // 父分组数据
private HashMap<String, List<String>> mChildData; // 子分组数据
public ExpandableListAdapter(Context context, List<String> groupTitles, HashMap<String, List<String>> childData) {
mContext = context;
mGroupTitles = groupTitles;
mChildData = childData;
}
@Override
public int getGroupCount() {
return mGroupTitles.size();
}
@Override
public int getChildrenCount(int groupPosition) {
return mChildData.get(mGroupTitles.get(groupPosition)).size();
}
@Override
public Object getGroup(int groupPosition) {
return mGroupTitles.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return mChildData.get(mGroupTitles.get(groupPosition)).get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
LayoutInflater inflater = (LayoutInflater) mContext.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = inflater.inflate(R.layout.list_item_group, null);
TextView title = (TextView) view.findViewById(R.id.group_title);
title.setText(mGroupTitles.get(groupPosition));
return view;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
LayoutInflater inflater = (LayoutInflater) mContext.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = inflater.inflate(R.layout.list_item_child, null);
TextView title = (TextView) view.findViewById(R.id.child_title);
title.setText(mChildData.get(mGroupTitles.get(groupPosition)).get(childPosition));
return view;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
```
2. 定义布局文件
list_item_group.xml
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:paddingLeft="40dp"
android:paddingRight="40dp"
android:paddingTop="20dp"
android:paddingBottom="20dp">
<TextView
android:id="@+id/group_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp" />
</LinearLayout>
```
list_item_child.xml
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:paddingLeft="40dp"
android:paddingRight="40dp"
android:paddingTop="15dp"
android:paddingBottom="15dp">
<TextView
android:id="@+id/child_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp" />
</LinearLayout>
```
3. 在 Activity 中使用 ExpandableListView
```
public class MainActivity extends AppCompatActivity {
private ExpandableListView mExpandableListView;
private ExpandableListAdapter mAdapter;
private List<String> mGroupTitles;
private HashMap<String, List<String>> mChildData;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化数据
initData();
// 初始化 ExpandableListView
mExpandableListView = (ExpandableListView) findViewById(R.id.expandable_list_view);
mAdapter = new ExpandableListAdapter(this, mGroupTitles, mChildData);
mExpandableListView.setAdapter(mAdapter);
// 设置子项点击监听器
mExpandableListView.setOnChildClickListener(new ExpandableListView.OnChildClickListener() {
@Override
public boolean onChildClick(ExpandableListView parent, View v, int groupPosition, int childPosition, long id) {
Toast.makeText(MainActivity.this, "您点击了" + mChildData.get(mGroupTitles.get(groupPosition)).get(childPosition), Toast.LENGTH_SHORT).show();
return true;
}
});
}
private void initData() {
mGroupTitles = new ArrayList<>();
mGroupTitles.add("计算机");
mGroupTitles.add("自然科学");
mGroupTitles.add("哲学");
List<String> computers = new ArrayList<>();
computers.add("Java");
computers.add("Python");
computers.add("C++");
computers.add("PHP");
computers.add("JavaScript");
computers.add("HTML/CSS");
computers.add("SQL");
List<String> sciences = new ArrayList<>();
sciences.add("物理");
sciences.add("化学");
sciences.add("生物");
sciences.add("地理");
sciences.add("天文");
List<String> philosophies = new ArrayList<>();
philosophies.add("伦理学");
philosophies.add("形而上学");
philosophies.add("认识论");
philosophies.add("逻辑学");
philosophies.add("美学");
mChildData = new HashMap<>();
mChildData.put(mGroupTitles.get(0), computers);
mChildData.put(mGroupTitles.get(1), sciences);
mChildData.put(mGroupTitles.get(2), philosophies);
}
}
```
这样,您就可以展示一个带父分组和子分组的 ExpandableListView ,同时设置子项点击监听器,实现相应的功能了。
阅读全文
相关推荐
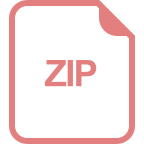
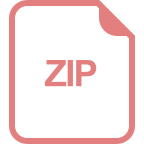
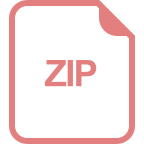
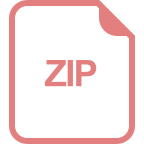
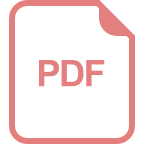
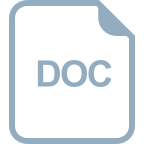
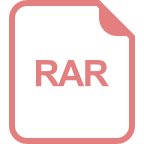
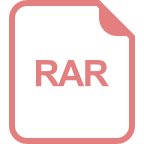
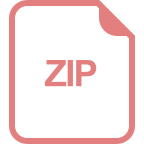
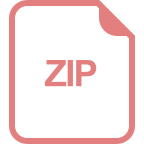
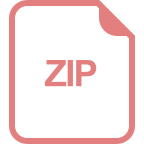
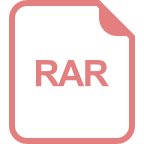
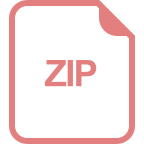
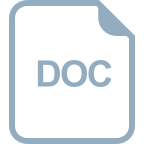
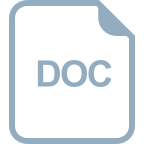
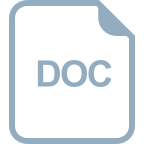