用java编写一段代码,可以实现定时自动点击按钮
时间: 2023-09-11 14:05:16 浏览: 233
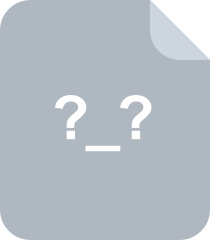
java 自动点击功能的实现

### 回答1:
可以使用java的Robot类来实现定时自动点击按钮,示例代码如下:
Robot robot = new Robot();
robot.delay(2000); // 延时2秒
robot.mouseMove(x, y); // 将鼠标移动到指定坐标
robot.mousePress(InputEvent.BUTTON1_MASK); // 模拟鼠标按下左键
robot.mouseRelease(InputEvent.BUTTON1_MASK); // 模拟鼠标释放左键
### 回答2:
使用Java编写代码实现定时自动点击按钮可以借助Java的图形用户界面(GUI)库和定时任务库。
首先,可以使用Java的GUI库如Swing或JavaFX来创建一个可视化窗口,其中包含一个按钮,用于模拟点击操作。
接着,使用Java的定时任务库,如java.util.Timer或ScheduledExecutorService,创建一个定时任务。设定定时任务的执行时间间隔,以及要执行的任务。
在定时任务中,使用Java的模拟点击方法,模拟按钮点击操作。可以使用Java的Robot类来实现模拟鼠标点击或键盘事件。例如,可以使用Robot类的mousePress和mouseRelease方法模拟鼠标点击按钮的位置。
下面是一个简单示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Timer;
import java.util.TimerTask;
public class AutoClickButton {
public static void main(String[] args) {
JFrame frame = new JFrame("Auto Click Button");
JButton button = new JButton("Click me");
frame.getContentPane().add(button);
frame.pack();
frame.setVisible(true);
// 创建一个定时任务
TimerTask task = new TimerTask() {
@Override
public void run() {
// 模拟点击按钮操作
try {
Robot robot = new Robot();
Point point = button.getLocationOnScreen();
int x = point.x + button.getWidth() / 2;
int y = point.y + button.getHeight() / 2;
robot.mouseMove(x, y);
robot.mousePress(InputEvent.BUTTON1_MASK);
robot.mouseRelease(InputEvent.BUTTON1_MASK);
} catch (AWTException e) {
e.printStackTrace();
}
}
};
// 创建一个定时器
Timer timer = new Timer();
// 设定定时任务执行的时间间隔,这里设定为每10秒执行一次
long delay = 0;
long period = 10000;
// 启动定时器
timer.schedule(task, delay, period);
// 监听按钮点击事件
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked");
}
});
}
}
```
这段代码创建了一个可视化窗口,包含一个按钮。然后使用定时任务每隔10秒模拟点击按钮操作。同时也监听了按钮的点击事件。
请注意,这只是一个简单的示例,具体的实现方式可能会因为使用不同的GUI库和定时任务库而有所不同。
### 回答3:
可以使用Java的AWT和Robot库来实现定时自动点击按钮的功能。下面是一个简单的示例代码:
```java
import java.awt.AWTException;
import java.awt.Robot;
import java.awt.event.InputEvent;
public class AutoClickButton {
public static void main(String[] args) {
try {
// 创建一个Robot实例
Robot robot = new Robot();
// 设置点击按钮的坐标(具体坐标根据实际情况进行调整)
int buttonX = 100;
int buttonY = 100;
// 设置定时点击的间隔时间(单位:毫秒)
int interval = 2000;
// 循环执行定时点击按钮操作
while (true) {
// 将鼠标移动到按钮位置
robot.mouseMove(buttonX, buttonY);
// 按下和释放鼠标左键
robot.mousePress(InputEvent.BUTTON1_DOWN_MASK);
robot.mouseRelease(InputEvent.BUTTON1_DOWN_MASK);
// 休眠设置的间隔时间
Thread.sleep(interval);
}
} catch (AWTException | InterruptedException e) {
e.printStackTrace();
}
}
}
```
这段代码使用Robot类模拟鼠标点击按钮的动作。程序会不停地移动鼠标到指定位置并模拟点击鼠标左键,然后等待指定的时间间隔后再次点击。你可以根据实际情况调整按钮的坐标和点击间隔时间。
请注意,在使用`Thread.sleep(interval)`时,需要处理`InterruptedException`异常。
阅读全文
相关推荐
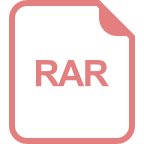
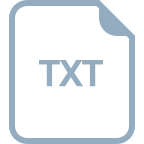
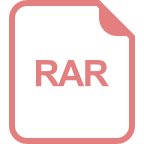
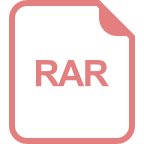
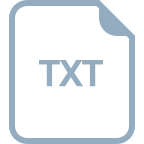
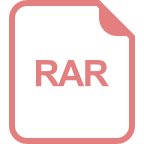
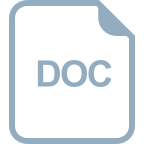
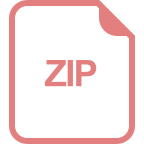
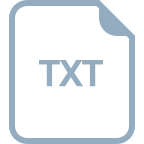
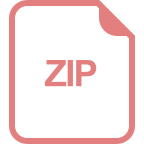
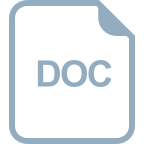
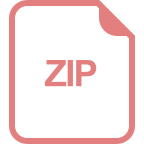
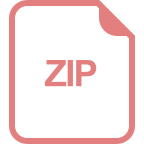
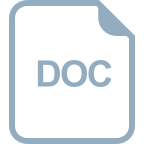
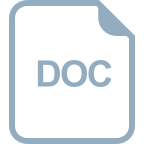
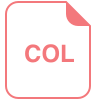