c语言顺序栈入栈出栈操作,输出一个数n,再输入n个字符,将每个字符依次入栈,再依次将字符出栈。
时间: 2024-09-29 17:09:28 浏览: 28
在C语言中,我们可以使用数组来模拟顺序栈(也称为简单堆栈)的入栈(push)和出栈(pop)操作。下面是一个简单的实现:
首先,我们需要定义一个栈结构体,包含一个字符数组`stack`以及栈顶指针top。
```c
#include <stdio.h>
typedef struct {
char stack[100]; // 简单栈,假设最大容量为99
int top;
} Stack;
// 入栈操作
void push(Stack *s, char c) {
if (s->top == sizeof(s->stack) - 1) {
printf("Stack is full, cannot push more elements.\n");
return;
}
s->stack[s->top] = c;
s->top++;
}
// 出栈操作
char pop(Stack *s) {
if (s->top == 0) {
printf("Stack is empty, no element to pop.\n");
return '\0'; // 返回空字符表示栈空
}
char c = s->stack[s->top - 1];
s->top--;
return c;
}
int main() {
Stack s;
int n;
printf("Enter the number of characters to be pushed: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
char input_char;
printf("Enter character %d: ", i + 1);
scanf(" %c", &input_char); // 注意这里加上了空格防止scanf读取多余的空白字符
push(&s, input_char);
}
for (int i = 0; i < n; i++) {
char output_char = pop(&s);
printf("Popped character: %c\n", output_char);
}
return 0;
}
```
在这个示例中,我们首先输入一个数字n,然后读取并入栈n个字符。之后,我们将栈中的字符依次弹出并打印。如果在入栈或出栈过程中遇到栈满或栈空的情况,会相应地给出错误提示。
相关推荐
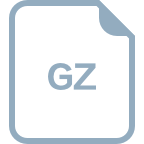
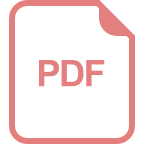
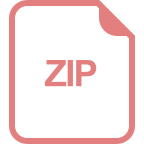














