word2vec对英语单词进行训练的python代码
时间: 2023-03-28 12:01:10 浏览: 103
以下是使用Python进行word2vec训练的示例代码:
```python
from gensim.models import Word2Vec
sentences = [['this', 'is', 'the', 'first', 'sentence', 'for', 'word2vec'],
['this', 'is', 'the', 'second', 'sentence'],
['yet', 'another', 'sentence'],
['one', 'more', 'sentence'],
['and', 'the', 'final', 'sentence']]
model = Word2Vec(sentences, min_count=1)
print(model)
```
这段代码使用gensim库中的Word2Vec模型对给定的句子进行训练,并打印出训练后的模型。
相关问题
word2vec关键词提取 python_如何从word2vec的Google预训练模型中提取单词向量?
要从Google预训练的word2vec模型中提取单词向量,可以使用Gensim库中的KeyedVectors类。以下是一个简单的Python代码示例:
```python
import gensim.downloader as api
# 加载预训练模型
model = api.load('word2vec-google-news-300')
# 获取单词向量
vector = model['word']
```
这里的`word`是你要获取向量的单词。如果你要获取多个单词的向量,可以将它们放在一个列表中并使用`model[]`方法一次获取它们的向量。
还要注意,这个预训练模型非常大,需要下载几个G的数据。另外,这个模型只提供了英语单词的向量,如果你需要处理其他语言的单词,你可能需要找到其他的预训练模型。
阅读全文
相关推荐
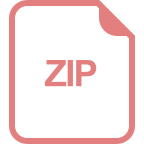
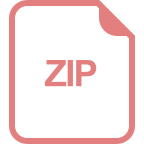
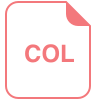
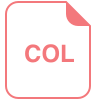
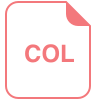
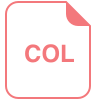
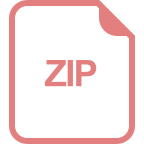
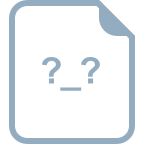
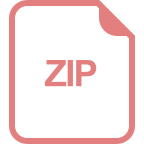
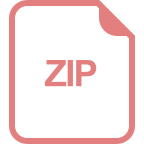
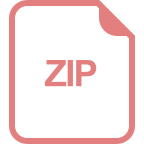
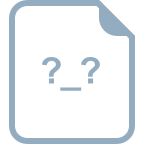
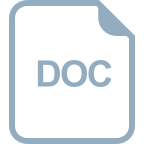
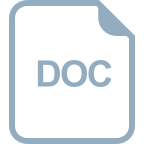
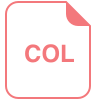
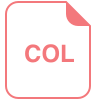