給我bert的範例code
时间: 2023-07-29 20:06:06 浏览: 104
好的,以下是BERT模型在PyTorch中的一个简单示例代码:
```python
import torch
from transformers import BertTokenizer, BertModel
# 加载预训练的BERT模型和tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased')
# 准备输入数据
text = "Hello, how are you today?"
tokens = tokenizer.tokenize(text)
input_ids = tokenizer.convert_tokens_to_ids(tokens)
input_tensor = torch.tensor([input_ids])
# 使用BERT模型进行前向传递
with torch.no_grad():
outputs = model(input_tensor)
# 获取BERT模型的输出
last_hidden_states = outputs[0]
```
在上面的示例中,我们使用`BertTokenizer`将输入文本转换为BERT模型接受的输入格式,并使用`BertModel`执行前向传递。最后,我们可以从模型输出中获取BERT模型的最后一层隐藏状态,该状态通常用于下游任务的输入。
相关问题
AttributeError: 'Adapter' object has no attribute 'init_bert_weights'
这个错误通常是因为你的代码中的 `Adapter` 类没有实现 `init_bert_weights` 方法。`init_bert_weights` 方法是在 `transformers` 库中的 `BertModel` 类中定义的,如果你想使用这个方法,你需要继承 `BertModel` 类并在你的适配器类中实现这个方法。以下是一个简单的例子:
```python
from transformers import BertModel
class MyAdapter(BertModel):
def __init__(self, config):
super().__init__(config)
# your adapter model layers here
def init_bert_weights(self, module):
# your initialization code here
```
在这个例子中,`MyAdapter` 继承了 `BertModel` 类,并实现了自己的适配器模型层。`init_bert_weights` 方法被定义在 `BertModel` 中,所以我们在 `MyAdapter` 中需要实现这个方法。你可以在这个方法中添加你自己的参数初始化代码,以便将 `BertModel` 的参数初始化为你的适配器模型所需的形状和值。
如果你不需要使用 `init_bert_weights` 方法,也可以从 `transformers` 库中导入 `Adapter` 类并使用它来构建你的适配器模型,例如:
```python
from transformers import Adapter
class MyAdapter(Adapter):
def __init__(self, config):
super().__init__(config)
# your adapter model layers here
```
这个适配器类不需要实现 `init_bert_weights` 方法,因为它已经被 `Adapter` 类实现了。
阅读全文
相关推荐
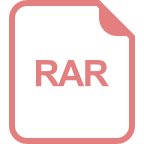
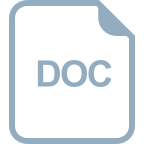
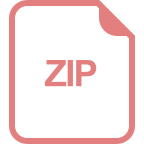
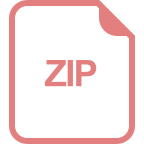
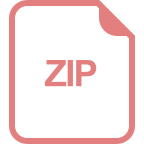
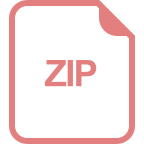
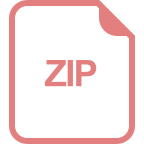
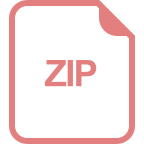
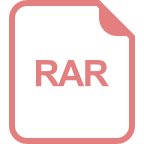
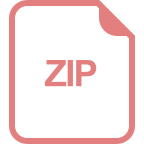