python贪吃蛇代码
时间: 2023-09-14 11:12:04 浏览: 59
以下是一个简单的 Python 贪吃蛇代码示例:
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 定义屏幕尺寸和背景颜色
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
BG_COLOR = (0, 0, 0)
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 设置窗口标题
pygame.display.set_caption('贪吃蛇')
# 定义贪吃蛇的初始位置和大小
snake_pos = [100, 50]
snake_body = [[100, 50], [90, 50], [80, 50]]
# 定义食物的初始位置和大小
food_pos = [random.randrange(1, SCREEN_WIDTH // 10) * 10,
random.randrange(1, SCREEN_HEIGHT // 10) * 10]
food_size = [10, 10]
# 定义贪吃蛇的移动速度和方向
snake_speed = 10
snake_dir = "RIGHT"
# 创建时钟对象,用于控制游戏帧率
clock = pygame.time.Clock()
# 定义游戏循环标志
game_over = False
# 游戏循环
while not game_over:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and snake_dir != "DOWN":
snake_dir = "UP"
elif event.key == pygame.K_DOWN and snake_dir != "UP":
snake_dir = "DOWN"
elif event.key == pygame.K_LEFT and snake_dir != "RIGHT":
snake_dir = "LEFT"
elif event.key == pygame.K_RIGHT and snake_dir != "LEFT":
snake_dir = "RIGHT"
# 移动贪吃蛇的身体
if snake_dir == "UP":
snake_pos[1] -= snake_speed
elif snake_dir == "DOWN":
snake_pos[1] += snake_speed
elif snake_dir == "LEFT":
snake_pos[0] -= snake_speed
elif snake_dir == "RIGHT":
snake_pos[0] += snake_speed
# 判断贪吃蛇是否吃到食物
if snake_pos[0] == food_pos[0] and snake_pos[1] == food_pos[1]:
food_pos = [random.randrange(1, SCREEN_WIDTH // 10) * 10,
random.randrange(1, SCREEN_HEIGHT // 10) * 10]
snake_body.append([snake_pos[0], snake_pos[1]])
# 绘制游戏背景
screen.fill(BG_COLOR)
# 绘制食物
pygame.draw.rect(screen, (255, 0, 0), pygame.Rect(
food_pos[0], food_pos[1], food_size[0], food_size[1]))
# 绘制贪吃蛇的身体
for pos in snake_body:
pygame.draw.rect(screen, (0, 255, 0), pygame.Rect(
pos[0], pos[1], 10, 10))
# 更新贪吃蛇身体位置
snake_body.insert(0, list(snake_pos))
snake_body.pop()
# 判断贪吃蛇是否碰到边界或自身
if snake_pos[0] < 0 or snake_pos[0] > SCREEN_WIDTH-10:
game_over = True
elif snake_pos[1] < 0 or snake_pos[1] > SCREEN_HEIGHT-10:
game_over = True
for block in snake_body[1:]:
if snake_pos[0] == block[0] and snake_pos[1] == block[1]:
game_over = True
# 刷新屏幕
pygame.display.update()
# 控制游戏帧率
clock.tick(20)
# 退出 Pygame 库
pygame.quit()
```
阅读全文
相关推荐
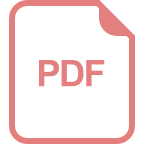


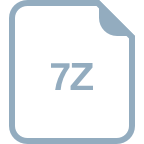
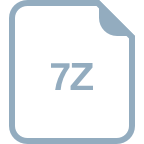
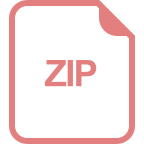
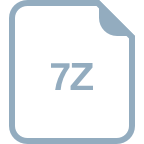
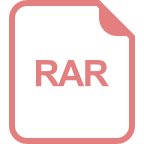
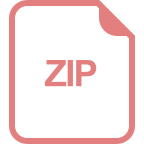
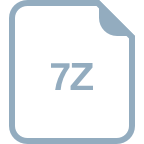