c语言写一个图书管理系统
时间: 2023-09-07 17:11:03 浏览: 48
好的,下面是一个简单的图书管理系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
typedef struct {
char title[50];
char author[50];
int year;
} Book;
Book library[MAX_BOOKS];
int num_books = 0;
void add_book() {
if (num_books == MAX_BOOKS) {
printf("Error: Library is full!\n");
return;
}
printf("Enter book title: ");
scanf("%s", library[num_books].title);
printf("Enter book author: ");
scanf("%s", library[num_books].author);
printf("Enter book year: ");
scanf("%d", &library[num_books].year);
num_books++;
printf("Book added successfully.\n");
}
void list_books() {
if (num_books == 0) {
printf("Library is empty.\n");
return;
}
printf("List of books:\n");
for (int i = 0; i < num_books; i++) {
printf("%d. %s by %s (%d)\n", i+1, library[i].title, library[i].author, library[i].year);
}
}
void search_book() {
if (num_books == 0) {
printf("Library is empty.\n");
return;
}
char search_title[50];
printf("Enter book title to search: ");
scanf("%s", search_title);
for (int i = 0; i < num_books; i++) {
if (strcmp(search_title, library[i].title) == 0) {
printf("%s by %s (%d)\n", library[i].title, library[i].author, library[i].year);
return;
}
}
printf("Book not found.\n");
}
int main() {
int choice;
do {
printf("\nLibrary Management System\n");
printf("1. Add book\n");
printf("2. List books\n");
printf("3. Search book\n");
printf("4. Quit\n");
printf("Enter choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
list_books();
break;
case 3:
search_book();
break;
case 4:
printf("Goodbye!\n");
exit(0);
default:
printf("Invalid choice. Try again.\n");
break;
}
} while (choice != 4);
return 0;
}
```
这个程序定义了 `Book` 结构体,其中包括书籍的标题、作者和出版年份。然后定义了一个 `library` 全局数组,用于存储图书馆中的图书。在主函数中,通过一个循环菜单,用户可以选择添加新书、列出所有书籍或搜索特定书籍。程序还包括一些基本的错误检查,例如防止图书馆已满或在搜索时不存在书籍。
相关推荐
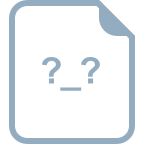
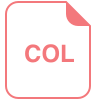


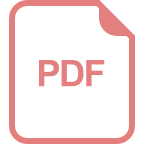
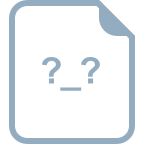
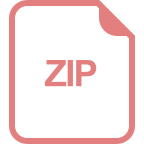
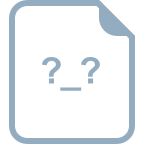
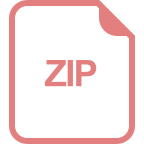
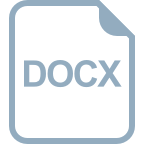
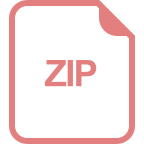