数组对象去除相同的属性名
时间: 2023-10-11 12:05:52 浏览: 49
你好!关于你的问题,可以使用 JavaScript 的 Set 数据结构和数组的 filter 方法来实现,具体操作如下:
```javascript
// 定义一个数组对象
const arr = [
{ id: 1, name: 'Tom', age: 20 },
{ id: 2, name: 'Jerry', age: 22 },
{ id: 3, name: 'Tom', gender: 'male' }
];
// 取出所有属性名
const keys = Array.from(new Set(arr.flatMap(obj => Object.keys(obj))));
// 去除相同属性名
const res = arr.map(obj =>
keys.reduce((acc, key) => {
if (obj.hasOwnProperty(key)) {
acc[key] = obj[key];
}
return acc;
}, {})
);
// 输出结果
console.log(res);
```
以上代码中,我们首先使用 Set 数据结构取出数组对象中所有属性名,并通过 flatMap 方法将它们打平为一个一维数组。然后使用 reduce 方法遍历数组对象,只取出原始对象中存在的属性名,最终返回一个新的对象数组。
相关问题
vue 两个数组对象相同名字去重
可以使用 JavaScript 的 `filter()` 和 `some()` 方法来实现。
假设有两个数组 `arr1` 和 `arr2`,每个数组中包含多个对象,对象有一个属性名为 `name`。
```javascript
const arr1 = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Mike' },
{ id: 3, name: 'Jane' }
];
const arr2 = [
{ id: 4, name: 'Jane' },
{ id: 5, name: 'David' },
{ id: 6, name: 'John' }
];
```
我们需要去重后的数组 `result`,其中包含两个原数组中所有不重复的对象。
```javascript
const result = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Mike' },
{ id: 3, name: 'Jane' },
{ id: 5, name: 'David' }
];
```
可以通过以下代码实现:
```javascript
// 合并两个数组
const allArr = [...arr1, ...arr2];
// 去重,保留第一个出现的对象
const result = allArr.filter((obj, index, self) => {
return index === self.findIndex(item => item.name === obj.name);
});
```
这里的 `filter()` 方法会遍历每个对象,保留第一个出现的同名对象,去除后续的同名对象。`some()` 方法用于查找是否有同名对象。
数组去重去掉数组内每一个对象的相同属性
好的,针对您的问题,可以使用 JavaScript 中的 reduce 和 findIndex 方法来实现。具体实现如下:
假设我们有一个数组 arr,每个对象都有属性 a 和 b,我们需要根据属性 a 进行去重,并且在去重后,每个对象的属性 b 不能相同。
```
const arr = [
{a: 1, b: 'apple'},
{a: 2, b: 'banana'},
{a: 1, b: 'peach'},
{a: 3, b: 'apple'},
{a: 1, b: 'orange'}
];
const result = arr.reduce((acc, cur) => {
const index = acc.findIndex(item => item.a === cur.a);
if (index === -1) {
acc.push(cur);
} else if (acc[index].b !== cur.b) {
acc[index].b = `${acc[index].b} and ${cur.b}`;
}
return acc;
}, []);
console.log(result);
// Output: [
// {a: 1, b: 'apple and peach and orange'},
// {a: 2, b: 'banana'},
// {a: 3, b: 'apple'}
// ]
```
以上代码中,我们使用 reduce 方法对数组进行遍历和操作。在每一次遍历中,我们使用 findIndex 方法来查找是否存在属性 a 相同的对象,如果不存在,则将当前对象添加到结果数组 acc 中。如果存在,我们需要判断当前对象的属性 b 是否与已存在对象的属性 b 相同,如果不同,则将其合并起来。
最终我们得到的结果为:
```
[
{a: 1, b: 'apple and peach and orange'},
{a: 2, b: 'banana'},
{a: 3, b: 'apple'}
]
```
相关推荐
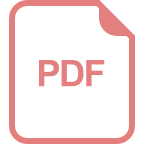
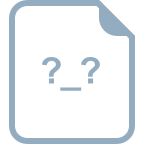
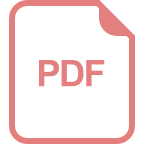
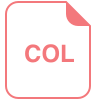
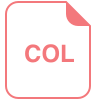










