编制课程管理系统C++
时间: 2023-07-11 08:32:23 浏览: 52
编制课程管理系统需要考虑以下几个方面:
1. 学生信息管理:包括学生的姓名、学号、性别、出生日期、班级等基本信息,可以使用结构体或类来管理学生信息。
2. 课程信息管理:包括课程的名称、课程号、学分、授课教师、上课时间等基本信息,同样可以使用结构体或类来管理课程信息。
3. 成绩管理:包括学生的课程成绩,可以使用二维数组或者链表来存储。
4. 界面设计:需要设计一个用户友好的界面,方便用户进行各种操作。
下面是一个简单的C++程序示例,实现了学生信息管理和课程信息管理:
```
#include <iostream>
#include <string>
using namespace std;
// 学生结构体
struct Student {
string name; // 学生姓名
int id; // 学号
string gender; // 性别
string birthday; // 出生日期
string class_name; // 班级
};
// 课程结构体
struct Course {
string name; // 课程名称
string id; // 课程号
int credit; // 学分
string teacher; // 授课教师
string time; // 上课时间
};
int main() {
// 定义学生数组和课程数组
const int MAX_STUDENT_NUM = 100;
const int MAX_COURSE_NUM = 20;
Student students[MAX_STUDENT_NUM];
Course courses[MAX_COURSE_NUM];
// 添加学生信息
Student s1 = {"张三", 1001, "男", "1999-01-01", "计算机科学与技术1班"};
Student s2 = {"李四", 1002, "女", "1999-02-02", "计算机科学与技术2班"};
students[0] = s1;
students[1] = s2;
// 添加课程信息
Course c1 = {"计算机组成原理", "C001", 3, "王五", "周一、周三 1-2节"};
Course c2 = {"操作系统", "C002", 4, "赵六", "周二、周四 3-4节"};
courses[0] = c1;
courses[1] = c2;
// 输出学生信息和课程信息
cout << "学生信息:" << endl;
for (int i = 0; i < 2; i++) {
cout << students[i].name << "\t" << students[i].id << "\t" << students[i].gender << "\t" << students[i].birthday << "\t" << students[i].class_name << endl;
}
cout << endl;
cout << "课程信息:" << endl;
for (int i = 0; i < 2; i++) {
cout << courses[i].name << "\t" << courses[i].id << "\t" << courses[i].credit << "\t" << courses[i].teacher << "\t" << courses[i].time << endl;
}
return 0;
}
```
这个程序实现了学生信息和课程信息的添加和输出。你可以在此基础上进一步完善,添加成绩管理和界面设计等功能。
相关推荐
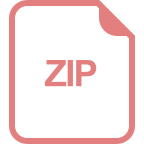
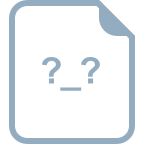
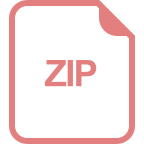














