分别使用WordNet算法和Lesk算法进行英文消歧义的Python实现
时间: 2024-03-05 11:53:35 浏览: 62
WordNet算法和Lesk算法都是用于英文消歧义的算法,它们可以帮助我们确定一个单词在特定上下文中的含义。下面是两种算法的Python实现:
1. WordNet算法实现:
```
from nltk.corpus import wordnet
def wordnet_disambiguate(word, sentence):
# 获取单词的同义词集
synsets = wordnet.synsets(word)
best_sense = None
max_overlap = 0
context = set(sentence)
for synset in synsets:
# 获取同义词集的定义和例句
definition = set(synset.definition().split())
examples = set(" ".join(synset.examples()).split())
# 计算上下文与定义和例句的重叠度
overlap = len(context.intersection(definition)) + len(context.intersection(examples))
# 选择最佳的同义词集
if overlap > max_overlap:
max_overlap = overlap
best_sense = synset
return best_sense.definition()
sentence = "I went to the bank to deposit my money."
word = "bank"
print(wordnet_disambiguate(word, sentence.split()))
```
2. Lesk算法实现:
```
from nltk.corpus import wordnet
from nltk.corpus import stopwords
def lesk_disambiguate(word, sentence):
# 获取单词的同义词集
synsets = wordnet.synsets(word)
best_sense = None
max_overlap = 0
context = set(sentence)
for synset in synsets:
# 获取同义词集的定义和例句
definition = set(synset.definition().split())
examples = set(" ".join(synset.examples()).split())
# 去除停用词
stop_words = set(stopwords.words('english'))
definition = definition.difference(stop_words)
examples = examples.difference(stop_words)
# 计算上下文与定义和例句的重叠度
overlap = len(context.intersection(definition)) + len(context.intersection(examples))
# 选择最佳的同义词集
if overlap > max_overlap:
max_overlap = overlap
best_sense = synset
return best_sense.definition()
sentence = "I went to the bank to deposit my money."
word = "bank"
print(lesk_disambiguate(word, sentence.split()))
```
这两种算法的实现过程大致相同,不同之处在于Lesk算法会去除停用词。在使用时只需传入待消歧义的单词和句子即可。
相关推荐
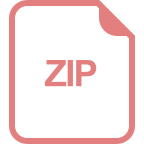
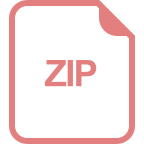
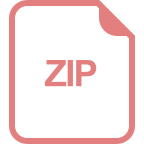














