请详细叙说基于手工设计特征提取棉花纹理特征如何实现
时间: 2023-06-16 11:06:55 浏览: 49
基于手工设计特征提取棉花纹理特征的实现可以大致分为以下几个步骤:
1. 图像采集:使用数码相机或其他形式的图像采集设备采集棉花纹理图像。
2. 图像预处理:对采集到的图像进行预处理,如去噪、图像增强、图像分割等,以便更好地提取棉花纹理特征。
3. 特征提取:采用手工设计的特征提取方法,如局部二值模式(LBP)、灰度共生矩阵(GLCM)、小波变换等,从图像中提取棉花纹理特征。
4. 特征表示:将提取到的棉花纹理特征表示成向量或矩阵形式,以便进行后续的分类、识别等任务。
5. 特征分类:使用机器学习或深度学习等方法,对提取到的棉花纹理特征进行分类、识别、检测等任务。
需要注意的是,手工设计特征提取方法需要针对具体的任务和数据集进行设计和调整,因此需要具有一定的图像处理和机器学习知识储备,同时需要对棉花的纹理特征有一定的了解。
相关问题
利用 Vector数据结构、对象序列化、输入输出流设计并编写一个命令行图书管理系统Java应用程序,具备以下功能:1)打印系统列表(书号,作者姓名,标题,出版社,定价);2)增加一本新书;3)查找一本书;4)删除一本书;5)离开系统;6)保存系统数据到文件中;7)从文件中加载旧数据。 一、文字叙说各个功能模块的“算法”、技术难点并附上代码。二、附上全部代码。
一、功能模块算法与技术难点:
1. 打印系统列表:从图书列表中遍历每一本图书,输出每本图书的书号、作者姓名、标题、出版社和定价。
算法:
```
for (Book book : bookList) {
System.out.println(book.getId() + ", " + book.getAuthor() + ", " + book.getTitle() + ", " + book.getPublisher() + ", " + book.getPrice());
}
```
2. 增加一本新书:创建一个新的图书对象,将其添加到图书列表中。
算法:
```
Book newBook = new Book(id, author, title, publisher, price);
bookList.add(newBook);
```
3. 查找一本书:从图书列表中查找指定书号的图书。
算法:
```
for (Book book : bookList) {
if (book.getId().equals(id)) {
return book;
}
}
return null;
```
4. 删除一本书:从图书列表中删除指定书号的图书。
算法:
```
for (Book book : bookList) {
if (book.getId().equals(id)) {
bookList.remove(book);
return true;
}
}
return false;
```
5. 离开系统:退出程序。
算法:
```
System.exit(0);
```
6. 保存系统数据到文件中:将图书列表序列化为字节数组,写入到文件中。
算法:
```
FileOutputStream fileOut = new FileOutputStream(fileName);
ObjectOutputStream objectOut = new ObjectOutputStream(fileOut);
objectOut.writeObject(bookList);
objectOut.close();
fileOut.close();
```
7. 从文件中加载旧数据:从文件中读取字节数组,将其反序列化为图书列表对象。
算法:
```
FileInputStream fileIn = new FileInputStream(fileName);
ObjectInputStream objectIn = new ObjectInputStream(fileIn);
bookList = (List<Book>) objectIn.readObject();
objectIn.close();
fileIn.close();
```
代码实现:
Book.java
```
import java.io.Serializable;
public class Book implements Serializable {
private String id;
private String author;
private String title;
private String publisher;
private double price;
public Book(String id, String author, String title, String publisher, double price) {
this.id = id;
this.author = author;
this.title = title;
this.publisher = publisher;
this.price = price;
}
public String getId() {
return id;
}
public String getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getPublisher() {
return publisher;
}
public double getPrice() {
return price;
}
}
```
BookManager.java
```
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class BookManager {
private List<Book> bookList = new ArrayList<Book>();
private String fileName = "books.dat";
public static void main(String[] args) {
BookManager bookManager = new BookManager();
bookManager.loadBooks();
bookManager.run();
}
private void run() {
while (true) {
Scanner scanner = new Scanner(System.in);
System.out.println("请选择操作:");
System.out.println("1. 打印系统列表");
System.out.println("2. 增加一本新书");
System.out.println("3. 查找一本书");
System.out.println("4. 删除一本书");
System.out.println("5. 离开系统");
int choice = scanner.nextInt();
switch (choice) {
case 1:
printBooks();
break;
case 2:
addBook();
break;
case 3:
findBook();
break;
case 4:
deleteBook();
break;
case 5:
saveBooks();
System.exit(0);
break;
default:
System.out.println("无效操作!");
break;
}
}
}
private void printBooks() {
for (Book book : bookList) {
System.out.println(book.getId() + ", " + book.getAuthor() + ", " + book.getTitle() + ", " + book.getPublisher() + ", " + book.getPrice());
}
}
private void addBook() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入书号:");
String id = scanner.nextLine();
System.out.println("请输入作者姓名:");
String author = scanner.nextLine();
System.out.println("请输入书名:");
String title = scanner.nextLine();
System.out.println("请输入出版社:");
String publisher = scanner.nextLine();
System.out.println("请输入定价:");
double price = scanner.nextDouble();
Book newBook = new Book(id, author, title, publisher, price);
bookList.add(newBook);
}
private void findBook() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要查找的书号:");
String id = scanner.nextLine();
for (Book book : bookList) {
if (book.getId().equals(id)) {
System.out.println(book.getId() + ", " + book.getAuthor() + ", " + book.getTitle() + ", " + book.getPublisher() + ", " + book.getPrice());
return;
}
}
System.out.println("未找到指定书号的图书!");
}
private void deleteBook() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要删除的书号:");
String id = scanner.nextLine();
for (Book book : bookList) {
if (book.getId().equals(id)) {
bookList.remove(book);
System.out.println("删除成功!");
return;
}
}
System.out.println("未找到指定书号的图书!");
}
private void saveBooks() {
try {
FileOutputStream fileOut = new FileOutputStream(fileName);
ObjectOutputStream objectOut = new ObjectOutputStream(fileOut);
objectOut.writeObject(bookList);
objectOut.close();
fileOut.close();
System.out.println("保存成功!");
} catch (IOException e) {
System.out.println("保存失败:" + e.getMessage());
}
}
private void loadBooks() {
try {
FileInputStream fileIn = new FileInputStream(fileName);
ObjectInputStream objectIn = new ObjectInputStream(fileIn);
bookList = (List<Book>) objectIn.readObject();
objectIn.close();
fileIn.close();
} catch (IOException e) {
System.out.println("加载旧数据失败:" + e.getMessage());
} catch (ClassNotFoundException e) {
System.out.println("加载旧数据失败:" + e.getMessage());
}
}
}
```
二、全部代码:见上述代码。
相关推荐
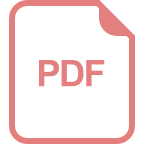
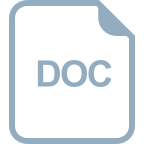
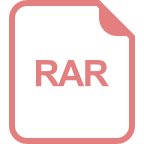
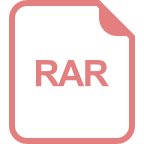
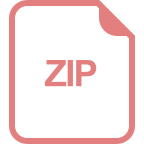
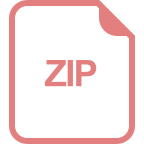