java时间段的查询_JAVA实现按时间段查询数据操作
时间: 2024-01-09 15:04:54 浏览: 46
在Java中实现按时间段查询数据操作,可以使用Java日期类(Date类、Calendar类等)和数据库查询语句结合使用。
首先需要构造时间段的起始时间和结束时间,可以使用Date类来表示时间。例如:
```
Date startDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse("2021-01-01 00:00:00");
Date endDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse("2021-01-31 23:59:59");
```
接下来使用SQL语句进行查询操作,例如MySQL数据库中的SELECT语句:
```
SELECT * FROM table_name WHERE date_column BETWEEN start_date AND end_date;
```
其中,table_name为要查询的表名,date_column为要查询的时间列名,start_date和end_date为时间段的起始时间和结束时间,可以使用Java中的SimpleDateFormat类将Date类型的时间转换为字符串类型的时间。
完整的代码示例:
```java
import java.sql.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class QueryByTime {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载驱动类
Class.forName("com.mysql.cj.jdbc.Driver");
// 获取数据库连接
String url = "jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 构造时间段的起始时间和结束时间
Date startDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse("2021-01-01 00:00:00");
Date endDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse("2021-01-31 23:59:59");
// 构造SQL语句
String sql = "SELECT * FROM table_name WHERE date_column BETWEEN ? AND ?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setTimestamp(1, new Timestamp(startDate.getTime()));
pstmt.setTimestamp(2, new Timestamp(endDate.getTime()));
// 执行查询操作
rs = pstmt.executeQuery();
// 处理查询结果
while (rs.next()) {
// TODO: 处理查询结果
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭资源
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
需要注意的是,上述代码的时间段起始时间和结束时间的格式必须与数据库中存储的时间格式一致,否则会查询不到数据。另外,还需要根据具体的数据库类型和版本来使用相应的JDBC驱动和连接方式。
相关推荐
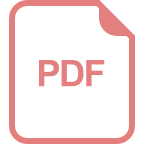
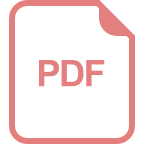
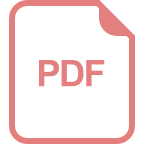
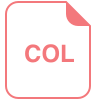













