请给我一个多标签时序二分类问题的代码,数据包含id号,时间序列,四项int输入,由csv读取。
时间: 2023-10-16 14:08:10 浏览: 45
这里给出一个基于PyTorch的多标签时序二分类问题的代码示例,数据包含id号,时间序列,四项int输入,由csv读取。假设要预测两个标签,即二分类问题。
首先,我们需要安装必要的库:`pandas`、`numpy`、`torch`、`torchvision`、`sklearn`。
```python
# 导入必要的库
import pandas as pd
import numpy as np
import torch
import torch.nn as nn
import torch.utils.data as Data
from sklearn.model_selection import train_test_split
from sklearn.metrics import classification_report
```
然后,我们读取数据并进行预处理。假设数据文件名为 'data.csv',其中标签列名分别为 'label1' 和 'label2'。
```python
# 读取数据
data = pd.read_csv('data.csv')
# 提取标签
labels = data[['label1', 'label2']].values
# 提取时间序列和四项int输入
time_series = data['time_series'].apply(lambda x: np.fromstring(x, sep=',')).values
inputs = data[['input1', 'input2', 'input3', 'input4']].values
# 划分训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(
np.concatenate((time_series.reshape(-1, 1), inputs), axis=1),
labels,
test_size=0.2,
random_state=42
)
```
接下来,我们需要定义一个数据集类,将数据转化为 PyTorch 中的 Dataset 类型。
```python
class MyDataset(Data.Dataset):
def __init__(self, x, y):
self.x = torch.from_numpy(x).float()
self.y = torch.from_numpy(y).float()
def __getitem__(self, index):
return self.x[index], self.y[index]
def __len__(self):
return len(self.x)
```
然后,我们定义一个多标签时序二分类模型。这里我们使用一个简单的 LSTM 模型。
```python
class Model(nn.Module):
def __init__(self):
super(Model, self).__init__()
self.lstm = nn.LSTM(input_size=6, hidden_size=64, num_layers=2, batch_first=True)
self.fc1 = nn.Linear(64, 32)
self.fc2 = nn.Linear(32, 2)
def forward(self, x):
out, _ = self.lstm(x)
out = out[:, -1, :]
out = nn.functional.relu(self.fc1(out))
out = self.fc2(out)
return out
```
接下来,我们定义一个训练函数和一个测试函数。
```python
def train(model, train_loader, optimizer):
model.train()
for batch_x, batch_y in train_loader:
optimizer.zero_grad()
output = model(batch_x)
loss = nn.BCEWithLogitsLoss()(output, batch_y)
loss.backward()
optimizer.step()
def test(model, test_loader):
model.eval()
y_true, y_pred = [], []
with torch.no_grad():
for batch_x, batch_y in test_loader:
output = model(batch_x)
y_true.append(batch_y.cpu().numpy())
y_pred.append(torch.sigmoid(output).cpu().numpy().round())
y_true = np.concatenate(y_true, axis=0)
y_pred = np.concatenate(y_pred, axis=0)
report = classification_report(y_true, y_pred)
print(report)
```
最后,我们可以进行模型的训练和测试。
```python
# 定义超参数
batch_size = 128
epochs = 10
lr = 0.001
# 定义数据集和数据加载器
train_dataset = MyDataset(x_train, y_train)
train_loader = Data.DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
test_dataset = MyDataset(x_test, y_test)
test_loader = Data.DataLoader(test_dataset, batch_size=batch_size)
# 初始化模型和优化器
model = Model()
optimizer = torch.optim.Adam(model.parameters(), lr=lr)
# 训练模型
for epoch in range(epochs):
train(model, train_loader, optimizer)
test(model, test_loader)
```
我们可以根据实际情况修改模型和超参数,以达到更好的性能。
相关推荐
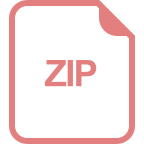
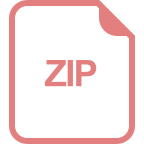
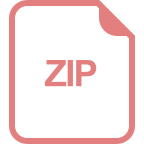
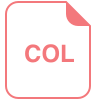
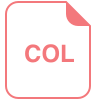
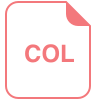
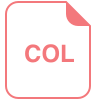
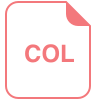







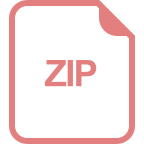
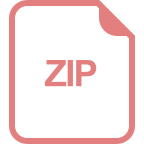
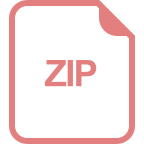