用python画奥特曼
时间: 2023-09-17 12:06:07 浏览: 397
### 回答1:
好的,下面是一份可以用Python绘制奥特曼的示例代码:
```python
from turtle import *
# 设置画笔的颜色和大小
pensize(10)
pencolor("silver")
# 绘制奥特曼的身体
begin_fill()
fillcolor("red")
circle(100)
end_fill()
# 移动画笔位置,绘制奥特曼的头部
penup()
goto(0, 200)
pendown()
begin_fill()
fillcolor("silver")
circle(50)
end_fill()
# 计算奥特曼眼睛和嘴巴的位置
eye_left_x, eye_left_y = -30, 240
eye_right_x, eye_right_y = 30, 240
mouth_x, mouth_y = 0, 210
# 移动画笔位置,绘制奥特曼的眼睛和嘴巴
penup()
goto(eye_left_x, eye_left_y)
pendown()
dot(20, "white")
penup()
goto(eye_right_x, eye_right_y)
pendown()
dot(20, "white")
penup()
goto(mouth_x, mouth_y)
pendown()
seth(-90)
circle(30, 180)
# 移动画笔位置,绘制奥特曼的手臂
penup()
goto(-130, 0)
pendown()
seth(-70)
forward(100)
penup()
goto(130, 0)
pendown()
seth(-110)
forward(100)
# 移动画笔位置,绘制奥特曼的腿
penup()
goto(-50, -200)
pendown()
seth(-90)
forward(100)
penup()
goto(50, -200)
pendown()
seth(-90)
forward(100)
# 隐藏画笔,完成绘制
hideturtle()
done()
```
运行代码后,将弹出一个窗口,显示绘制的奥特曼图像。如果需要保存图像,可以使用Turtle提供的`getcanvas()`方法获取画布对象,然后使用画布对象的`postscript()`方法将图像保存为PostScript文件,最后使用Pillow库将PostScript文件转换为其他格式的图像。
### 回答2:
要使用Python绘制奥特曼,可以使用图形库turtle来创建绘图窗口和绘制的图形。以下是一个简单的示例代码:
```python
import turtle
def draw_ultraman():
# 创建绘图窗口
window = turtle.Screen()
window.bgcolor("white")
# 创建绘图对象
pen = turtle.Turtle()
# 设置绘图对象的属性
pen.speed(3) # 设置绘制速度
# 绘制奥特曼的头部
pen.fillcolor("red") # 设置填充颜色为红色
pen.begin_fill() # 开始填充
pen.circle(100) # 绘制圆形头部
pen.end_fill() # 结束填充
# 绘制奥特曼的身体
pen.up() # 抬起画笔
pen.goto(0, -100) # 移动到身体位置
pen.down() # 落下画笔
pen.fillcolor("silver") # 设置填充颜色为银色
pen.begin_fill() # 开始填充
pen.circle(150) # 绘制圆形身体
pen.end_fill() # 结束填充
# 绘制奥特曼的眼睛
pen.up() # 抬起画笔
pen.goto(-60, 60) # 移动到左眼位置
pen.down() # 落下画笔
pen.fillcolor("white") # 设置填充颜色为白色
pen.begin_fill() # 开始填充
pen.circle(30) # 绘制左眼
pen.end_fill() # 结束填充
pen.up() # 抬起画笔
pen.goto(60, 60) # 移动到右眼位置
pen.down() # 落下画笔
pen.fillcolor("white") # 设置填充颜色为白色
pen.begin_fill() # 开始填充
pen.circle(30) # 绘制右眼
pen.end_fill() # 结束填充
# 绘制奥特曼的嘴巴
pen.up() # 抬起画笔
pen.goto(-30, -30) # 移动到嘴巴位置
pen.down() # 落下画笔
pen.width(5) # 设置画笔宽度为5
pen.circle(30, 180) # 绘制半圆形嘴巴
# 绘制奥特曼的红线图案
pen.up() # 抬起画笔
pen.goto(-150, -100) # 移动到开始绘制红线的位置
pen.down() # 落下画笔
pen.color("red") # 设置画笔颜色为红色
pen.width(10) # 设置画笔宽度为10
pen.goto(150, -100) # 绘制红线
# 隐藏绘图对象
pen.hideturtle()
# 关闭绘图窗口
window.exitonclick()
# 调用函数绘制奥特曼
draw_ultraman()
```
这段代码使用了turtle库中的函数来绘制奥特曼的头部、身体、眼睛、嘴巴和红线图案。可以根据需要对绘制的形状、颜色和位置进行调整。最后调用`window.exitonclick()`可以点击窗口来关闭绘图窗口。
### 回答3:
要用Python画奥特曼,我们可以使用Python的图像处理库来实现。
首先,我们需要导入所需的库,包括`PIL`(Python Imaging Library)和`numpy`(用于处理图像数据):
```python
from PIL import Image, ImageDraw
import numpy as np
```
接下来,我们创建一个画布,设置好奥特曼的头部、身体、手臂、腿部的形状和颜色:
```python
canvas = Image.new('RGB', (500, 500), 'white')
draw = ImageDraw.Draw(canvas)
head_color = (219, 47, 52)
body_color = (0, 38, 109)
arm_color = (0, 38, 109)
leg_color = (0, 38, 109)
```
然后,我们使用`draw.polygon`函数来绘制奥特曼的头部、身体、手臂、腿部:
```python
# 头部
head_points = np.array([[150, 50], [350, 50], [400, 150], [350, 250], [150, 250], [100, 150]])
draw.polygon(list(map(tuple, head_points)), fill=head_color)
# 身体
body_points = np.array([[200, 250], [200, 450], [300, 450], [300, 250]])
draw.polygon(list(map(tuple, body_points)), fill=body_color)
# 左手臂
arm_points = np.array([[100, 250], [200, 200], [200, 250]])
draw.polygon(list(map(tuple, arm_points)), fill=arm_color)
# 右手臂
arm_points = np.array([[300, 200], [400, 250], [400, 250], [300, 250]])
draw.polygon(list(map(tuple, arm_points)), fill=arm_color)
# 左腿
leg_points = np.array([[225, 450], [275, 450], [275, 500], [225, 500]])
draw.polygon(list(map(tuple, leg_points)), fill=leg_color)
# 右腿
leg_points = np.array([[225, 450], [225, 500], [175, 500], [175, 450]])
draw.polygon(list(map(tuple, leg_points)), fill=leg_color)
```
最后,保存绘制的奥特曼图像,并展示出来:
```python
canvas.save('Ultraman.png')
canvas.show()
```
这样,我们就可以通过运行这段代码来使用Python绘制一个奥特曼的图像并保存为`Ultraman.png`文件。
阅读全文
相关推荐














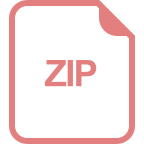