帮我用Java写一份飞行棋代码
时间: 2024-01-26 16:02:09 浏览: 125
好的,以下是一个简单的飞行棋游戏的Java代码。这里只提供基本的游戏逻辑,UI部分需要自行完成。
```java
import java.util.Scanner;
public class FlightChess {
public static final int PLAYER_NUM = 4; // 玩家数量
public static final int MAP_SIZE = 56; // 地图格子数量
public static final int FINISH_LINE = 50; // 终点线格子数
public static final int[] DICE_NUMS = {1, 2, 3, 4, 5, 6}; // 骰子数字
public static void main(String[] args) {
// 初始化游戏
Player[] players = new Player[PLAYER_NUM];
for (int i = 0; i < PLAYER_NUM; i++) {
players[i] = new Player("Player " + (i + 1));
}
Map map = new Map(MAP_SIZE);
// 游戏主循环
int currentPlayerIndex = 0;
while (true) {
Player currentPlayer = players[currentPlayerIndex];
System.out.println("It's " + currentPlayer.getName() + "'s turn.");
// 掷骰子
System.out.println("Press Enter to roll the dice...");
Scanner scanner = new Scanner(System.in);
scanner.nextLine();
int diceNum = DICE_NUMS[(int)(Math.random() * DICE_NUMS.length)];
System.out.println("You rolled a " + diceNum + ".");
// 移动棋子
int oldPos = currentPlayer.getPos();
int newPos = oldPos + diceNum;
if (newPos >= FINISH_LINE) {
newPos = FINISH_LINE;
System.out.println(currentPlayer.getName() + " has reached the finish line!");
currentPlayer.setWin(true);
}
int[] obstaclePositions = map.getObstaclePositions();
for (int obstaclePos : obstaclePositions) {
if (newPos == obstaclePos) {
newPos = oldPos;
System.out.println("Oops, there's an obstacle at " + obstaclePos + "! You have to stay where you are.");
}
}
currentPlayer.setPos(newPos);
System.out.println(currentPlayer.getName() + " moved from " + oldPos + " to " + newPos + ".");
// 轮到下一个玩家
System.out.println();
currentPlayerIndex = (currentPlayerIndex + 1) % PLAYER_NUM;
if (currentPlayer.isWin()) {
break;
}
}
// 游戏结束
for (int i = 0; i < PLAYER_NUM; i++) {
if (players[i].isWin()) {
System.out.println(players[i].getName() + " wins!");
}
}
}
}
class Player {
private String name;
private int pos;
private boolean win;
public Player(String name) {
this.name = name;
this.pos = 0;
this.win = false;
}
public String getName() {
return name;
}
public int getPos() {
return pos;
}
public void setPos(int pos) {
this.pos = pos;
}
public boolean isWin() {
return win;
}
public void setWin(boolean win) {
this.win = win;
}
}
class Map {
private int size;
private int[] obstaclePositions;
public Map(int size) {
this.size = size;
this.obstaclePositions = generateObstaclePositions();
}
public int[] getObstaclePositions() {
return obstaclePositions;
}
private int[] generateObstaclePositions() {
int[] positions = new int[4];
for (int i = 0; i < 4; i++) {
int pos = (int)(Math.random() * size);
while (pos == 0 || pos == 14 || pos == 28 || pos == 42 || hasCollision(pos, positions, i)) {
pos = (int)(Math.random() * size);
}
positions[i] = pos;
}
return positions;
}
private boolean hasCollision(int pos, int[] positions, int count) {
for (int i = 0; i < count; i++) {
if (pos == positions[i]) {
return true;
}
}
return false;
}
}
```
这个代码实现了一个简单的飞行棋游戏,包括四个玩家,一个地图,随机生成的障碍物和一个终点线。玩家依次掷骰子,移动棋子,遇到障碍物则不能前进。当有玩家到达终点线时,游戏结束。
请注意,这份代码并不完美,可能需要根据实际需求进行修改和优化。
阅读全文
相关推荐
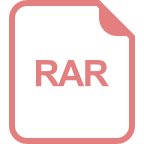
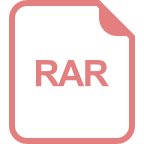
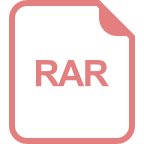
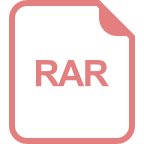
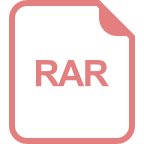

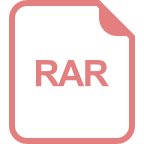
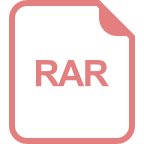

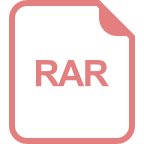
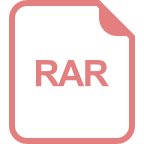
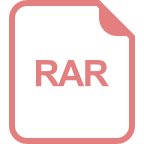