把数据activity传递到fragment
时间: 2023-11-19 21:12:22 浏览: 113
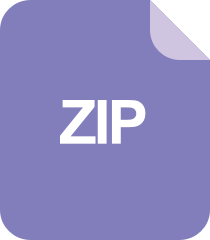
FragmentDemo4:Android-使用片段对象将数据从活动传递到片段
有多种方法可以将数据从Activity传递到Fragment:
1. Bundle
Activity可以使用Bundle将数据传递给Fragment。在Activity中创建Bundle对象,将数据添加到其中,然后使用setArguments()方法将Bundle对象传递给Fragment。在Fragment中,可以使用getArguments()方法获取传递的Bundle对象,并从中获取数据。
在Activity中:
```
Bundle bundle = new Bundle();
bundle.putString("key", "value");
MyFragment fragment = new MyFragment();
fragment.setArguments(bundle);
```
在Fragment中:
```
Bundle bundle = getArguments();
String value = bundle.getString("key");
```
2. 接口
Activity可以实现一个接口,该接口定义了一个方法,用于将数据传递给实现该接口的Fragment。在Fragment中,实现该接口,并在onAttach()方法中将Activity转换为该接口。然后,Activity可以调用该接口的方法,将数据传递给Fragment。
在Activity中:
```
public interface MyInterface {
void sendData(String data);
}
MyInterface myInterface;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
myInterface = new MyFragment();
}
public void sendDataToFragment(String data) {
myInterface.sendData(data);
}
```
在Fragment中:
```
public class MyFragment extends Fragment implements MainActivity.MyInterface {
@Override
public void onAttach(Context context) {
super.onAttach(context);
MainActivity activity = (MainActivity) context;
activity.setMyInterface(this);
}
@Override
public void sendData(String data) {
// 处理数据
}
}
```
3. ViewModel
使用ViewModel可以在Activity和Fragment之间共享数据。创建一个ViewModel类,在其中保存数据,然后在Activity和Fragment中都可以访问该ViewModel类,并获取或设置数据。
在ViewModel中:
```
public class MyViewModel extends ViewModel {
private MutableLiveData<String> data = new MutableLiveData<>();
public void setData(String value) {
data.setValue(value);
}
public LiveData<String> getData() {
return data;
}
}
```
在Activity中:
```
MyViewModel viewModel = ViewModelProviders.of(this).get(MyViewModel.class);
viewModel.setData("value");
```
在Fragment中:
```
MyViewModel viewModel = ViewModelProviders.of(getActivity()).get(MyViewModel.class);
viewModel.getData().observe(this, new Observer<String>() {
@Override
public void onChanged(String value) {
// 处理数据
}
});
```
阅读全文
相关推荐
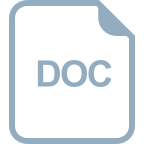
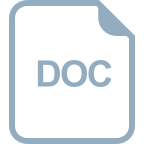
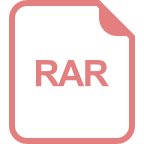
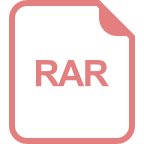
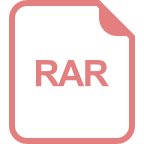
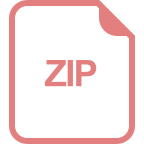
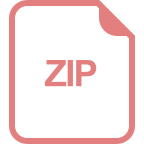
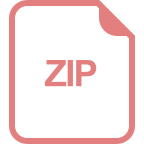
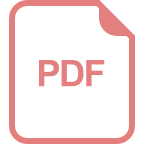








