如何将捕获到的数据包导入mysql数据库中,代码怎么实现,封装成函数
时间: 2024-01-22 14:18:52 浏览: 91
要将捕获到的数据包导入到MySQL数据库中,可以使用Python中的pcapy和pymysql模块。pcapy用于捕获网络数据包,pymysql用于连接和操作MySQL数据库。
以下是一个简单的将捕获到的数据包导入MySQL数据库的示例代码,可以根据自己的需要进行修改和扩展:
```python
import pcapy
import pymysql
# 连接MySQL数据库
conn = pymysql.connect(host='localhost',
port=3306,
user='root',
password='password',
db='test_db')
# 创建游标对象
cursor = conn.cursor()
# 定义插入数据的SQL语句
insert_sql = "INSERT INTO packet (timestamp, length, src_ip, dst_ip, protocol) VALUES (%s, %s, %s, %s, %s)"
# 定义捕获数据包的回调函数
def packet_handler(header, data):
# 解析数据包
timestamp = header.getts()[0]
length = header.getlen()
src_ip = ".".join(map(str, data[26:30]))
dst_ip = ".".join(map(str, data[30:34]))
protocol = data[23]
# 执行插入数据的SQL语句
cursor.execute(insert_sql, (timestamp, length, src_ip, dst_ip, protocol))
conn.commit()
# 打开网络接口并开始捕获数据包
cap = pcapy.open_live("eth0", 65536, True, 100)
# 捕获数据包并调用回调函数处理
cap.loop(-1, packet_handler)
# 关闭游标和数据库连接
cursor.close()
conn.close()
```
上述代码中,首先使用pymysql模块连接MySQL数据库,然后定义了一个插入数据的SQL语句。接着定义了一个捕获数据包的回调函数packet_handler,用于解析数据包并将数据插入到MySQL数据库中。最后使用pcapy模块打开网络接口并开始捕获数据包,将捕获到的数据包传递给回调函数进行处理。最后关闭游标和数据库连接。
将上述代码封装成函数的示例代码如下:
```python
import pcapy
import pymysql
def capture_and_save_to_mysql(interface, db_config, table_name):
# 连接MySQL数据库
conn = pymysql.connect(**db_config)
# 创建游标对象
cursor = conn.cursor()
# 定义插入数据的SQL语句
insert_sql = f"INSERT INTO {table_name} (timestamp, length, src_ip, dst_ip, protocol) VALUES (%s, %s, %s, %s, %s)"
# 定义捕获数据包的回调函数
def packet_handler(header, data):
# 解析数据包
timestamp = header.getts()[0]
length = header.getlen()
src_ip = ".".join(map(str, data[26:30]))
dst_ip = ".".join(map(str, data[30:34]))
protocol = data[23]
# 执行插入数据的SQL语句
cursor.execute(insert_sql, (timestamp, length, src_ip, dst_ip, protocol))
conn.commit()
# 打开网络接口并开始捕获数据包
cap = pcapy.open_live(interface, 65536, True, 100)
# 捕获数据包并调用回调函数处理
cap.loop(-1, packet_handler)
# 关闭游标和数据库连接
cursor.close()
conn.close()
```
使用示例:
```python
interface = 'eth0'
db_config = {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': 'password',
'db': 'test_db'
}
table_name = 'packet'
capture_and_save_to_mysql(interface, db_config, table_name)
```
在调用函数时,需要传入要捕获的网络接口名称、MySQL数据库连接配置和数据表名称作为参数。
阅读全文
相关推荐

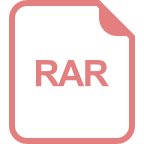
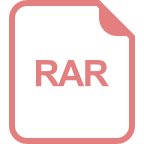
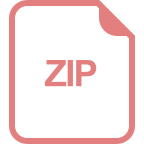
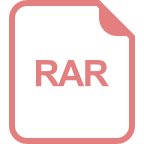
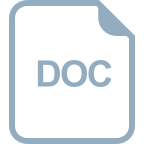
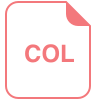
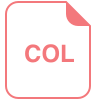
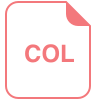
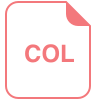
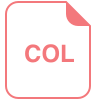
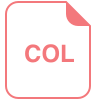
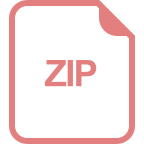