C++语言:输入一行字符,分别统计出其中的英文字母、空格、数字和其他字符的个数。
时间: 2023-11-07 10:57:22 浏览: 37
好的,这是一个计算问题,让我帮您解答。以下是 C 语言的代码:
```c
#include <stdio.h>
int main() {
char str[100];
int i, letters=0, space=0, digits=0, others=0;
printf("请输入一行字符:\n");
fgets(str, 100, stdin);
for(i=0; str[i]!='\0'; i++) {
if((str[i]>='a'&& str[i]<='z')||(str[i]>='A'&&str[i]<='Z')) {
letters++;
} else if(str[i]==' ') {
space++;
} else if(str[i]>='0'&&str[i]<='9') {
digits++;
} else {
others++;
}
}
printf("英文字母个数:%d\n", letters);
printf("空格个数:%d\n", space);
printf("数字个数:%d\n", digits);
printf("其他字符个数:%d\n", others);
return 0;
}
```
希望对您有帮助!
相关问题
使用c++编写:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。
使用C语言编写输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数的程序:#include <stdio.h>
int main()
{
char str[100];
int letter = 0, space = 0, digit = 0, other = 0;
gets(str);
int i = 0;
while(str[i] != '\0')
{
if((str[i]>='a' && str[i]<='z') || (str[i]>='A' && str[i]<='Z'))
{
letter++;
}
else if(str[i] >= '0' && str[i] <= '9')
{
digit++;
}
else if(str[i] == ' ')
{
space++;
}
else
{
other++;
}
i++;
}
printf("字母个数:%d\n", letter);
printf("空格个数:%d\n", space);
printf("数字个数:%d\n", digit);
printf("其它字符个数:%d\n", other);
return 0;
}
用c++语言输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数
### 回答1:
可以使用C++的标准库中的字符处理函数来实现。具体方法如下:
```c++
#include <iostream>
#include <cctype> // 包含字符处理函数的头文件
using namespace std;
int main() {
string str;
getline(cin, str); // 输入一行字符串
int letter = 0, space = 0, digit = 0, other = 0; // 分别记录字母、空格、数字和其它字符个数
for (int i = 0; i < str.length(); i++) {
if (isalpha(str[i])) {
letter++;
} else if (isspace(str[i])) {
space++;
} else if (isdigit(str[i])) {
digit++;
} else {
other++;
}
}
cout << "字母个数:" << letter << endl;
cout << "空格个数:" << space << endl;
cout << "数字个数:" << digit << endl;
cout << "其它字符个数:" << other << endl;
return 0;
}
```
这段代码中,首先使用`getline`函数输入一行字符串,然后用一个循环遍历字符串中的每个字符,使用`isalpha`、`isspace`和`isdigit`函数判断字符的类型,并分别进行计数。最后输出统计结果。
### 回答2:
以下是使用C语言编写的代码,用于输入一行字符并统计其中的英文字母、空格、数字和其他字符的个数:
```c
#include <stdio.h>
int main() {
char input[200];
int letterCount = 0;
int spaceCount = 0;
int digitCount = 0;
int otherCount = 0;
printf("请输入一行字符:");
fgets(input, sizeof(input), stdin);
for (int i = 0; input[i] != '\0'; i++) {
if ((input[i] >= 'a' && input[i] <= 'z') || (input[i] >= 'A' && input[i] <= 'Z')) {
letterCount++;
} else if (input[i] == ' ') {
spaceCount++;
} else if (input[i] >= '0' && input[i] <= '9') {
digitCount++;
} else {
otherCount++;
}
}
printf("输入的字符中,英文字母个数为:%d\n", letterCount);
printf("输入的字符中,空格个数为:%d\n", spaceCount);
printf("输入的字符中,数字个数为:%d\n", digitCount);
printf("输入的字符中,其他字符个数为:%d\n", otherCount);
return 0;
}
```
运行以上代码时,程序会提示输入一行字符。用户输入后,程序会对每个字符进行判断,并统计英文字母、空格、数字和其他字符的个数。最后,程序会输出各个类型字符的个数。
### 回答3:
问题的要求是使用C语言输入一行字符,然后统计其中英文字母、空格、数字和其他字符的个数。下面是解决该问题的一个简单实现:
```c
#include <stdio.h>
int main() {
char line[100];
int i, alphabets, digits, spaces, others;
// 读取一行字符
printf("请输入一行字符:");
fgets(line, sizeof(line), stdin);
// 初始化计数器
alphabets = digits = spaces = others = 0;
// 遍历字符数组进行统计
for (i = 0; line[i] != '\0'; i++) {
if ((line[i] >= 'a' && line[i] <= 'z') || (line[i] >= 'A' && line[i] <= 'Z')) {
alphabets++;
} else if (line[i] >= '0' && line[i] <= '9') {
digits++;
} else if (line[i] == ' ') {
spaces++;
} else {
others++;
}
}
// 打印统计结果
printf("英文字母个数:%d\n", alphabets);
printf("数字个数:%d\n", digits);
printf("空格个数:%d\n", spaces);
printf("其他字符个数:%d\n", others);
return 0;
}
```
在此实现中,我们使用了一个字符数组 `line` 来存储输入的一行字符。然后,通过 `fgets` 函数从标准输入读取一行字符。接着,我们初始化了 `alphabets`、`digits`、`spaces` 和 `others` 四个计数器,并利用一个循环来遍历字符数组进行统计。在遍历过程中,我们通过判断字符的 ASCII 值来确定其属于哪一类(英文字母、数字、空格或其他字符),然后递增相应的计数器。最后,我们打印出四个计数器的值,即统计结果。
相关推荐
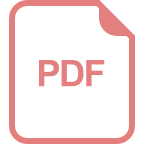
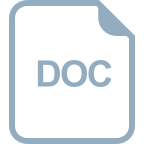












