打印杨辉三角c语言代码
时间: 2023-05-21 11:03:21 浏览: 139
以下是打印杨辉三角的 C 语言代码:
#include <stdio.h>
int main()
{
int rows, coef = 1, space, i, j;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for(i=0; i<rows; i++)
{
for(space=1; space <= rows-i; space++)
printf(" ");
for(j=0; j <= i; j++)
{
if (j==0 || i==0)
coef = 1;
else
coef = coef*(i-j+1)/j;
printf("%4d", coef);
}
printf("\n");
}
return 0;
}
相关问题
队列打印杨辉三角C语言代码
在C语言中,队列(Queue)通常用于处理需要先进先出(FIFO)的数据结构,但杨辉三角是一个典型的动态数组应用,它并不是直接与队列相关的。然而,如果你想要用C语言实现一个函数来打印杨辉三角,可以使用嵌套循环并存储每一行的数据在临时数组中,然后逐行输出,而不是真正意义上的队列操作。
下面是一个简单的C语言函数,用于打印杨辉三角:
```c
#include <stdio.h>
#include <stdlib.h>
void printPascalTriangle(int rows) {
int** triangle = (int**)malloc(rows * sizeof(int*));
for (int i = 0; i < rows; ++i) {
triangle[i] = (int*)malloc((i + 1) * sizeof(int));
triangle[i][0] = triangle[i][i] = 1;
}
for (int i = 0; i < rows; ++i) {
for (int j = 1; j < i; ++j) {
triangle[i][j] = triangle[i - 1][j - 1] + triangle[i - 1][j];
}
printf("%d ", triangle[i][0]);
for (int k = 1; k < i; ++k) {
printf("%d ", triangle[i][k]);
}
printf("\n");
}
// 释放内存
for (int i = 0; i < rows; ++i)
free(triangle[i]);
free(triangle);
}
int main() {
int rows;
printf("Enter the number of rows for Pascal's Triangle: ");
scanf("%d", &rows);
printPascalTriangle(rows);
return 0;
}
```
打印杨辉三角形C语言
以下是打印杨辉三角形的C语言代码:
```c
#include <stdio.h>
int main() {
int rows, coef = 1, space, i, j;
printf("请输入要打印的行数:"); scanf("%d", &rows);
for (i = 0; i < rows; i++) {
for (space = 1; space < rows - i; space++)
printf(" ");
for (j = 0; j <= i; j++) {
if (j == 0 || i == 0)
coef = 1;
else
coef = coef * (i - j + 1) / j;
printf("%-4d", coef);
}
printf("\n");
}
return 0;
}
```
运行结果如下:
```
请输入要打印的行数:6
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
```
阅读全文
相关推荐
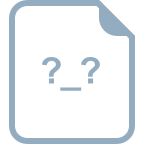
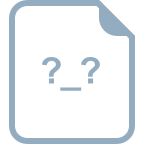
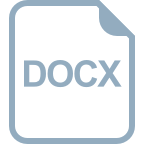
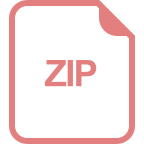








