javafx实现多选功能
时间: 2024-02-03 10:03:23 浏览: 24
JavaFX中实现多选功能可以使用CheckBox或者ListView来实现。
1. CheckBox实现多选功能:
CheckBox是JavaFX中提供的一个复选框控件,可以用来实现多选功能。下面是一个简单的示例代码:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class CheckBoxDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
VBox root = new VBox();
root.setSpacing(10);
root.setPadding(new Insets(10));
CheckBox cb1 = new CheckBox("选项1");
CheckBox cb2 = new CheckBox("选项2");
CheckBox cb3 = new CheckBox("选项3");
root.getChildren().addAll(cb1, cb2, cb3);
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
2. ListView实现多选功能:
ListView是JavaFX中提供的一个列表控件,可以用来实现多选功能。下面是一个简单的示例代码:
```java
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ListViewDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
VBox root = new VBox();
root.setSpacing(10);
root.setPadding(new Insets(10));
ObservableList<String> items = FXCollections.observableArrayList(
"选项1", "选项2", "选项3", "选项4", "选项5"
);
ListView<String> listView = new ListView<>(items);
listView.getSelectionModel().setSelectionMode(javafx.scene.control.SelectionMode.MULTIPLE);
root.getChildren().add(listView);
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在ListView中设置SelectionMode为MULTIPLE,即可实现多选功能。
相关推荐
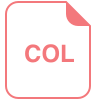













