请将上述代码改为可以随意输入任意英文明文的代码
时间: 2024-02-22 11:54:55 浏览: 55
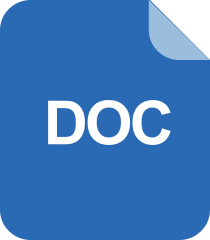
修改代码!!!
为了实现任意输入任意英文明文的代码,我们可以使用 `fgets` 函数从标准输入中读取明文。另外,为了避免输入的明文超过数组大小,我们可以使用动态内存分配来分配足够的空间。
下面是一份修改后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 密钥字法加密表
char key_table[26] = {'p', 'h', 'q', 'g', 'i', 'u', 'a', 'y', 'l', 'n', 'o', 'f', 'd', 'x', 'k', 'r', 'e', 's', 'z', 'v', 'c', 'm', 'j', 'b', 'w', 't'};
// 洗牌法加密表
int shuffle_table[26] = {23, 19, 11, 0, 7, 20, 2, 12, 6, 8, 16, 3, 15, 18, 1, 21, 5, 10, 25, 13, 14, 4, 22, 24, 9, 17};
// 公式法加密表
int formula_table[26] = {7, 14, 21, 4, 11, 18, 1, 8, 15, 22, 5, 12, 19, 2, 9, 16, 23, 6, 13, 20, 3, 10, 17, 24, 0, 25};
// 加密函数
void encrypt(char *plaintext, char *ciphertext, int len, char *key) {
int i, j;
char temp;
for (i = 0; i < len; i++) {
// 密钥字法加密
ciphertext[i] = key_table[key[i % strlen(key)] - 'a'];
// 洗牌法加密
for (j = 0; j < 26; j++) {
if (ciphertext[i] == j + 'a') {
ciphertext[i] = shuffle_table[j] + 'a';
break;
}
}
// 公式法加密
ciphertext[i] = (ciphertext[i] - 'a' + formula_table[i % 26]) % 26 + 'a';
// 对空格和标点符号不进行加密
if (plaintext[i] == ' ' || (plaintext[i] >= '0' && plaintext[i] <= '9') || (plaintext[i] >= 'a' && plaintext[i] <= 'z')) {
ciphertext[i] = plaintext[i];
}
}
ciphertext[len] = '\0';
}
// 解密函数
void decrypt(char *ciphertext, char *plaintext, int len, char *key) {
int i, j;
char temp;
for (i = 0; i < len; i++) {
// 公式法解密
plaintext[i] = (ciphertext[i] - 'a' - formula_table[i % 26] + 26) % 26 + 'a';
// 洗牌法解密
for (j = 0; j < 26; j++) {
if (plaintext[i] == shuffle_table[j] + 'a') {
plaintext[i] = j + 'a';
break;
}
}
// 密钥字法解密
for (j = 0; j < 26; j++) {
if (plaintext[i] == key_table[j]) {
plaintext[i] = 'a' + j;
break;
}
}
// 对空格和标点符号不进行解密
if (ciphertext[i] == ' ' || (ciphertext[i] >= '0' && ciphertext[i] <= '9') || (ciphertext[i] >= 'a' && ciphertext[i] <= 'z')) {
plaintext[i] = ciphertext[i];
}
}
plaintext[len] = '\0';
}
// 测试函数
int main() {
char key[] = "secret";
char *plaintext = NULL;
char *ciphertext = NULL;
char *decrypted = NULL;
int len;
printf("Please enter plaintext:\n");
fgets(plaintext, 100, stdin);
len = strlen(plaintext) - 1;
plaintext[len] = '\0';
ciphertext = (char*)malloc(len * sizeof(char));
decrypted = (char*)malloc(len * sizeof(char));
encrypt(plaintext, ciphertext, len, key);
printf("Encrypted text: %s\n", ciphertext);
decrypt(ciphertext, decrypted, len, key);
printf("Decrypted text: %s\n", decrypted);
free(plaintext);
free(ciphertext);
free(decrypted);
return 0;
}
```
在上述代码中,我们使用 `fgets` 函数从标准输入中读取明文,并使用动态内存分配来分配足够的空间。同时,我们在程序结束前需要释放这些空间。
阅读全文
相关推荐
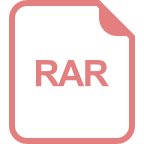
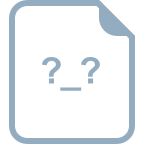
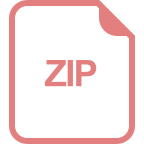
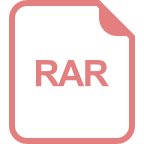
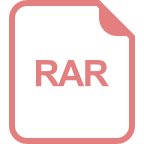
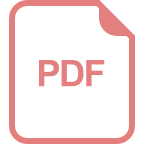
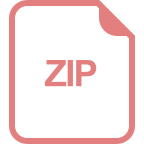
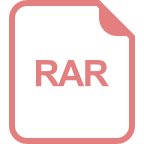
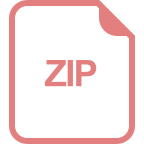
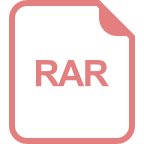
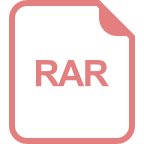
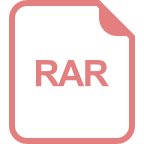
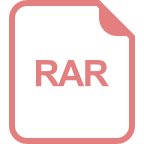