spring boot编写文件在线预览接口
时间: 2023-09-09 16:10:06 浏览: 56
以下是一个使用Spring Boot编写文件在线预览接口的示例:
1. 在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.21</version>
</dependency>
```
2. 编写一个控制器类,处理文件预览请求:
```java
@RestController
@RequestMapping("/preview")
public class PreviewController {
@GetMapping("/{fileName:.+}")
public ResponseEntity<byte[]> previewFile(@PathVariable String fileName) throws IOException {
String extension = FilenameUtils.getExtension(fileName);
MediaType mediaType = MediaType.APPLICATION_OCTET_STREAM;
if ("pdf".equalsIgnoreCase(extension)) {
mediaType = MediaType.APPLICATION_PDF;
} else if ("doc".equalsIgnoreCase(extension) || "docx".equalsIgnoreCase(extension)) {
mediaType = MediaType.APPLICATION_MSWORD;
} else if ("xls".equalsIgnoreCase(extension) || "xlsx".equalsIgnoreCase(extension)) {
mediaType = MediaType.APPLICATION_EXCEL;
} else if ("ppt".equalsIgnoreCase(extension) || "pptx".equalsIgnoreCase(extension)) {
mediaType = MediaType.APPLICATION_POWERPOINT;
}
HttpHeaders headers = new HttpHeaders();
headers.setContentType(mediaType);
headers.setContentDisposition(ContentDisposition.builder("inline").filename(fileName).build());
byte[] fileBytes = Files.readAllBytes(Paths.get(fileName));
return new ResponseEntity<>(fileBytes, headers, HttpStatus.OK);
}
}
```
3. 在`application.properties`文件中配置文件存储路径:
```properties
file.upload-dir=/path/to/uploaded/files/
```
4. 使用`MultipartFile`接收文件上传请求,保存文件到指定路径:
```java
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
String fileName = file.getOriginalFilename();
Path filePath = Paths.get(uploadDir + fileName);
Files.write(filePath, file.getBytes());
return ResponseEntity.ok("File uploaded successfully");
}
```
5. 在前端页面中使用`<iframe>`标签加载预览接口,例如:
```html
<iframe src="/preview/example.pdf" width="100%" height="600"></iframe>
```
注意:本示例中只实现了对PDF、Word、Excel和PowerPoint文件的预览,如果需要支持其他类型的文件,需要根据实际情况进行修改。
相关推荐
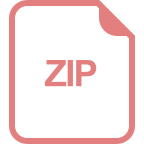
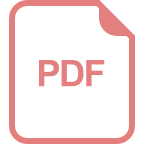














