用Java定义一个汽车类,要求有颜色、轮胎数量、速度等成员属性,并且汽车要有加速、减速、停车方法 定义一个小汽车类继承汽车类,重写相关方法,并添加载人方法,定义一个重卡类,继承汽车并重写 相关方法,并且还有载货方法,小汽车和重卡都有变形方法(接口)
时间: 2024-04-29 18:22:43 浏览: 9
汽车类:
```java
public class Car {
private String color;
private int numOfWheels;
private int speed;
public Car(String color, int numOfWheels, int speed) {
this.color = color;
this.numOfWheels = numOfWheels;
this.speed = speed;
}
public void accelerate(int amount) {
speed += amount;
}
public void decelerate(int amount) {
speed -= amount;
}
public void stop() {
speed = 0;
}
}
```
小汽车类:
```java
public class Car extends Vehicle {
private int numOfPassengers;
public Car(String color, int numOfWheels, int speed, int numOfPassengers) {
super(color, numOfWheels, speed);
this.numOfPassengers = numOfPassengers;
}
public void accelerate(int amount) {
if (speed + amount <= 120) {
super.accelerate(amount);
} else {
System.out.println("Cannot accelerate to that speed.");
}
}
public void decelerate(int amount) {
if (speed - amount >= 0) {
super.decelerate(amount);
} else {
System.out.println("Cannot decelerate to that speed.");
}
}
public void stop() {
super.stop();
numOfPassengers = 0;
}
public void carryPassengers(int numOfPassengers) {
this.numOfPassengers = numOfPassengers;
}
public void transform() {
System.out.println("The car has transformed into a robot!");
}
}
```
重卡类:
```java
public class Truck extends Vehicle {
private int maxCargoWeight;
public Truck(String color, int numOfWheels, int speed, int maxCargoWeight) {
super(color, numOfWheels, speed);
this.maxCargoWeight = maxCargoWeight;
}
public void accelerate(int amount) {
if (speed + amount <= 80) {
super.accelerate(amount);
} else {
System.out.println("Cannot accelerate to that speed.");
}
}
public void decelerate(int amount) {
if (speed - amount >= 0) {
super.decelerate(amount);
} else {
System.out.println("Cannot decelerate to that speed.");
}
}
public void stop() {
super.stop();
System.out.println("All cargo has been unloaded.");
}
public void carryCargo(int weight) {
if (weight <= maxCargoWeight) {
System.out.println("Cargo loaded.");
} else {
System.out.println("Cargo too heavy.");
}
}
public void transform() {
System.out.println("The truck has transformed into a giant robot!");
}
}
```
变形接口:
```java
public interface Transformable {
void transform();
}
```
相关推荐
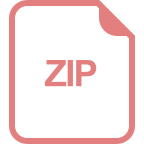
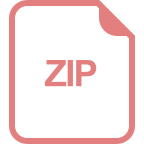















