使用_thread模块创建多线程的例子python
时间: 2023-11-22 16:53:11 浏览: 191
好的,以下是使用_thread模块创建多线程的python代码示例:
```
import _thread
# 定义线程函数
def worker(threadID, delay):
while True:
print("Thread ID {} is running".format(threadID))
time.sleep(delay)
# 创建线程
try:
_thread.start_new_thread(worker, (1, 1))
_thread.start_new_thread(worker, (2, 2))
_thread.start_new_thread(worker, (3, 3))
except:
print("Error: unable to start thread")
while True:
pass # 主线程无限运行
```
在这个例子中,我们定义了一个worker函数,它会在不断循环的过程中打印当前线程的ID,并且使用time模块中的sleep函数,让线程在打印之间暂停一段时间。接着我们使用_thread模块的start_new_thread函数来创建三个线程,并分别给它们传递不同的线程ID和延迟时间。最后,我们让主线程无限运行,以保证程序不会退出。
相关问题
使用 _thread 模块如何创建多线程,举个例子python
好的,您的问题是关于使用_thread模块创建多线程的例子。以下是一个使用_thread模块创建两个线程的例子:
```
import _thread
import time
# 定义一个函数,用于打印当前线程名字和时间
def print_time(threadName, delay):
count = 0
while count < 5:
time.sleep(delay)
count += 1
print("%s: %s" % (threadName, time.ctime(time.time())))
try:
# 创建线程1
_thread.start_new_thread( print_time, ("Thread-1", 2, ) )
# 创建线程2
_thread.start_new_thread( print_time, ("Thread-2", 4, ) )
except:
print("Error: 无法启动线程")
# 让主线程等待子线程结束
while 1:
pass
```
在上面的例子中,我们定义了一个名为print_time的函数,这个函数会打印当前线程的名字和当前时间,然后休眠一段时间。接下来,在try语句块中,我们使用_thread.start_new_thread()函数分别创建了两个线程,这两个线程都调用了print_time函数,并传入不同的线程名字和休眠时间。最后,我们让主线程等待子线程结束,以保证程序不会过早退出。
python 多线程例子
### 回答1:
下面是一个使用 Python 的 `threading` 模块创建多线程的简单示例:
```
import threading
def worker():
"""thread worker function"""
print('Worker')
threads = []
for i in range(5):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
```
这段代码会创建 5 个线程,每个线程都会运行 `worker` 函数。
还有一种使用Python的线程池的写法,使用concurrent.futures库中的ThreadPoolExecutor
```
import concurrent.futures
def worker():
"""thread worker function"""
print('Worker')
with concurrent.futures.ThreadPoolExecutor() as executor:
executor.map(worker, range(5))
```
这样可以更好的管理线程并发数,更方便的获取线程结果。
注意:请在你的程序中加入必要的同步操作,避免线程间数据竞争。
### 回答2:
Python多线程是指在一个程序中同时运行多个线程,每个线程执行不同的任务。通过多线程可以提高程序的运行时间和效率。
下面是一个简单的Python多线程例子:
```python
import threading
def print_numbers():
for i in range(1, 11):
print(i)
def print_letters():
for char in 'ABCDEFGHIJ':
print(char)
if __name__ == "__main__":
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print("程序结束")
```
在这个例子中,我们定义了两个函数`print_numbers`和`print_letters`,分别用于打印数字1到10和字母A到J。然后,我们使用`threading.Thread`类创建了两个线程:`thread1`和`thread2`,并通过`target`参数指定这两个线程分别执行`print_numbers`和`print_letters`。
接下来,我们使用`start()`方法启动这两个线程,并使用`join()`方法确保两个线程都执行完成。最后,我们打印出"程序结束",表示程序执行完毕。
运行这个程序时,会同时打印出数字和字母,而不是一个接一个地打印。这是因为两个线程是同时执行的,它们之间相互独立,互不干扰。
这就是一个简单的Python多线程例子,通过多线程可以有效地提高程序的执行效率。
### 回答3:
Python多线程可以通过使用线程类和线程函数来实现。以下是一个简单的示例:
1. 使用线程类:
import threading
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print("线程", self.name, "开始执行")
# 执行线程的任务
print("线程", self.name, "执行完毕")
# 创建线程对象
thread1 = MyThread("Thread-1")
thread2 = MyThread("Thread-2")
# 启动线程
thread1.start()
thread2.start()
# 等待线程结束
thread1.join()
thread2.join()
2. 使用线程函数:
import threading
def thread_function(name):
print("线程", name, "开始执行")
# 执行线程的任务
print("线程", name, "执行完毕")
# 创建线程对象
thread1 = threading.Thread(target=thread_function, args=("Thread-1",))
thread2 = threading.Thread(target=thread_function, args=("Thread-2",))
# 启动线程
thread1.start()
thread2.start()
# 等待线程结束
thread1.join()
thread2.join()
以上示例使用了threading模块中的Thread类和Thread函数来创建和启动线程。在线程类中,可以重写run方法来定义线程的任务;而在线程函数中,需要传入一个目标函数和参数来执行线程的任务。
注意,多线程并不一定就会提升性能,因为在Python中,全局解释器锁(GIL)会限制同一时间只有一个线程可以执行字节码指令。然而,在某些情况下,多线程仍然可以提高效率,如IO密集型任务。
阅读全文
相关推荐
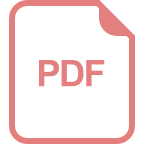
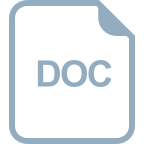
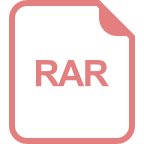
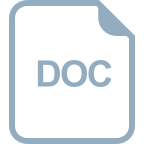
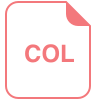

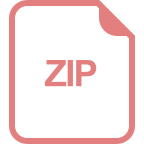
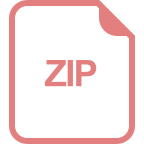
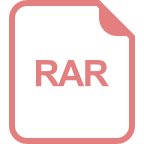
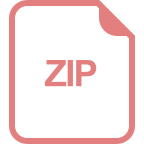
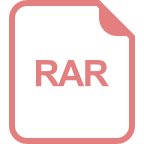
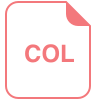

