Java启动一个rtsp服务,并创建一个连接
时间: 2024-01-13 11:05:53 浏览: 151
要启动一个RTSP服务并创建一个连接,您需要使用Java的RTSP客户端和服务器库。以下是一些步骤:
1. 下载并安装Java RTSP库,例如JRTPLIB或live555。
2. 编写一个Java程序来启动RTSP服务器,例如使用live555库中的“live555MediaServer”类。
3. 确定您要连接的RTSP服务器的IP地址和端口号。
4. 编写一个Java程序来连接RTSP服务器,例如使用JRTPLIB库中的“RTPSession”类。
5. 在连接成功后,您可以使用RTPSession类来发送和接收数据。
以下是一个简单的Java程序示例,用于启动一个RTSP服务器并创建一个连接:
```
import java.net.*;
import java.io.*;
public class RTSPClient {
public static void main(String[] args) {
try {
// Connect to RTSP server
Socket rtspSocket = new Socket("192.168.0.1", 554);
// Send RTSP request
OutputStream outputStream = rtspSocket.getOutputStream();
outputStream.write("DESCRIBE rtsp://192.168.0.1/stream.sdp RTSP/1.0\r\n".getBytes());
outputStream.write("CSeq: 1\r\n".getBytes());
outputStream.write("\r\n".getBytes());
// Receive RTSP response
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(rtspSocket.getInputStream()));
String response = bufferedReader.readLine();
while (response != null) {
System.out.println(response);
response = bufferedReader.readLine();
}
// Create RTP session
RTPSession rtpSession = new RTPSession("192.168.0.1", 1234);
// Send RTP packet
byte[] data = {1, 2, 3, 4, 5};
rtpSession.sendData(data);
// Receive RTP packet
byte[] buffer = new byte[1024];
int len = rtpSession.receiveData(buffer);
System.out.println("Received " + len + " bytes of data");
// Close RTP session and RTSP connection
rtpSession.endSession();
rtspSocket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
注意:这只是一个简单的示例程序,实际的实现可能需要更多的代码和配置。
阅读全文
相关推荐
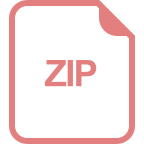
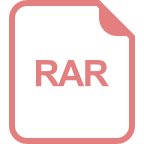

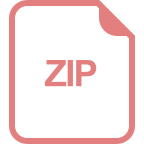
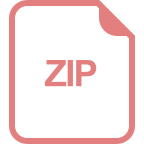
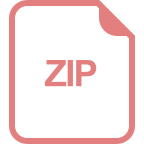
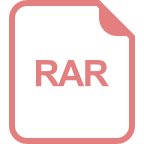
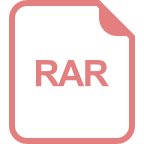
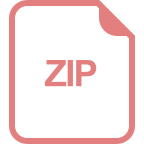
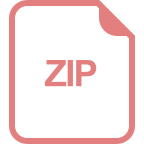
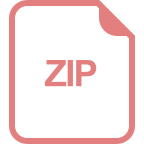
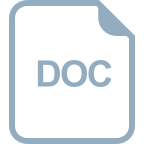
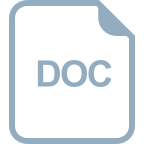
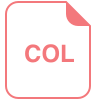
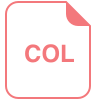


