用指向指针的指针的方法对5个字符串排序并输出
时间: 2023-04-28 08:01:16 浏览: 58
可以使用 qsort() 函数对指向指针的指针的数组进行排序。qsort() 函数需要比较函数作为参数。比较函数用于比较两个字符串的大小。
示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int compare(const void* a, const void* b) {
return strcmp(*(char**)a, *(char**)b);
}
int main() {
char* strings[] = {"apple", "banana", "cherry", "date", "elderberry"};
int n = sizeof(strings) / sizeof(strings[0]);
qsort(strings, n, sizeof(char*), compare);
for (int i = 0; i < n; i++) {
printf("%s\n", strings[i]);
}
return 0;
}
```
上面的代码定义了一个字符串数组,并使用 qsort() 函数对其进行排序。最后使用 for 循环输出排序后的字符串。
相关问题
用指向指针的指针方法对5个字符串排序并输出
这里提供一种用指向指针的指针方法对5个字符串排序并输出的示例代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void sortStrings(char** strings, int n) {
for (int i = ; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (strcmp(strings[i], strings[j]) > ) {
char* temp = strings[i];
strings[i] = strings[j];
strings[j] = temp;
}
}
}
}
int main() {
const int n = 5;
char* strings[n] = {"hello", "world", "apple", "banana", "orange"};
sortStrings(strings, n);
for (int i = ; i < n; i++) {
cout << strings[i] << endl;
}
return ;
}
```
这里定义了一个指向指针的指针 `char** strings`,它指向一个包含5个字符串的数组。在 `sortStrings` 函数中,使用了冒泡排序的方法对这5个字符串进行排序,最后在 `main` 函数中输出排序后的结果。
用指向指针的方法对5个字符串排序并输出
抱歉,作为AI语言模型,我无法直接执行代码。但是,我可以给您提供一个基本的思路:
1. 定义5个字符串数组,例如str1、str2、str3、str4、str5。
2. 定义一个指向指针的指针数组,例如char **strs。
3. 将5个字符串数组的地址存储到指针数组中,例如:
strs[0] = str1;
strs[1] = str2;
strs[2] = str3;
strs[3] = str4;
strs[4] = str5;
4. 使用冒泡排序或快速排序等算法对指针数组中的字符串进行排序。
5. 输出排序后的字符串,例如:
for (int i = 0; i < 5; i++) {
printf("%s\n", strs[i]);
}
希望这个思路能够帮助您完成题目要求。
相关推荐
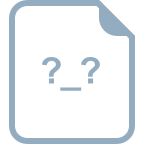
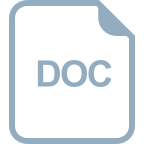












