python编程实现ECC的基本运算规则,用类定义点
时间: 2024-02-24 22:55:21 浏览: 146
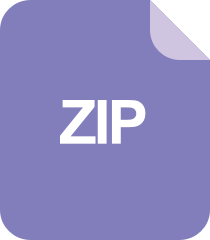
ecc的简单实现

下面是Python实现ECC基本运算规则,并用类定义点的示例代码:
```python
class Point:
def __init__(self, x, y, a, p_mod):
self.x = x
self.y = y
self.a = a
self.p_mod = p_mod
def __str__(self):
return f'({self.x},{self.y})'
def __eq__(self, other):
if isinstance(other, Point):
return self.x == other.x and self.y == other.y and self.a == other.a and self.p_mod == other.p_mod
return False
def point_add(self, other):
if self == other:
return self.point_double()
elif self.x is None:
return other
elif other.x is None:
return self
elif self.x == other.x and self.y != other.y:
return Point(None, None, self.a, self.p_mod)
else:
slope = None
if self.x == other.x:
slope = (3 * self.x * self.x + self.a) * pow(2 * self.y, -1, self.p_mod)
else:
slope = (other.y - self.y) * pow(other.x - self.x, -1, self.p_mod)
x = (slope * slope - self.x - other.x) % self.p_mod
y = (slope * (self.x - x) - self.y) % self.p_mod
return Point(x, y, self.a, self.p_mod)
def point_double(self):
if self.y == 0:
return Point(None, None, self.a, self.p_mod)
slope = (3 * self.x * self.x + self.a) * pow(2 * self.y, -1, self.p_mod)
x = (slope * slope - 2 * self.x) % self.p_mod
y = (slope * (self.x - x) - self.y) % self.p_mod
return Point(x, y, self.a, self.p_mod)
def point_multiply(self, n):
if n == 0 or self.x is None:
return Point(None, None, self.a, self.p_mod)
if n == 1:
return self
if n % 2 == 0:
return (self.point_double()).point_multiply(n // 2)
else:
return self.point_add(self.point_double().point_multiply((n - 1) // 2))
```
在以上代码中,我们首先定义了一个`Point`类,用于表示椭圆曲线上的一个点。`__init__`方法用于初始化点的坐标、曲线参数以及模数。`__str__`方法用于输出点的坐标。`__eq__`方法用于判断两个点是否相等。
接下来,我们定义了三个方法:`point_add`用于实现点加运算,`point_double`用于实现点倍运算,`point_multiply`用于实现点乘运算。
在上述方法中,我们使用了Python内置的`pow`函数求模数下的乘法逆元。注意,为了方便处理无穷远点,我们使用了`None`来表示无穷远点的坐标。
使用该类定义的点,我们可以方便地进行ECC基本运算,例如:
```python
p1 = Point(2, 7, 5, 23)
p2 = Point(-1, -5, 5, 23)
p3 = p1.point_add(p2)
print(p3) # 输出: (3, 1)
p4 = p1.point_multiply(3)
print(p4) # 输出: (13, 14)
```
阅读全文
相关推荐
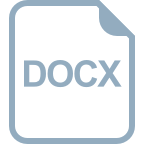
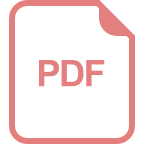



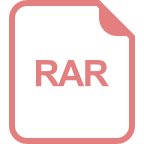
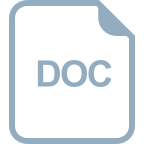
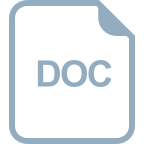
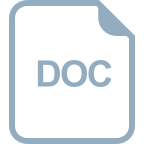
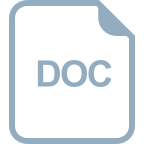
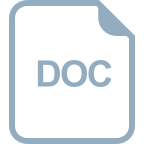
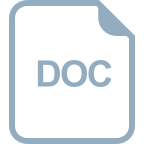
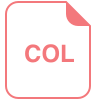
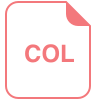
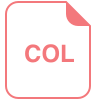
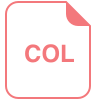
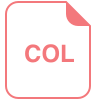
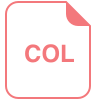