根据输入的灰度图像,分别计算图像的均值、方差等统计特征,并计算图像的直方图特征并以图形方式显示图像的直方图(用C或C++语言实现)不能用opencv和其他现成的库函数操作;。
时间: 2024-02-12 14:07:08 浏览: 110
好的,如果不能使用OpenCV等图像处理库函数,那么可以使用C或C++语言自己编写代码实现。以下是一种基本的实现方法:
```c++
#include <iostream>
#include <cmath>
const int MAX_VALUE = 255; // 灰度值的最大值
// 计算图像的均值和方差
void calc_mean_and_stddev(unsigned char* data, int width, int height, double& mean, double& stddev) {
double sum = 0, sum_sq = 0;
int count = width * height;
for (int i = 0; i < count; ++i) {
sum += data[i];
sum_sq += data[i] * data[i];
}
mean = sum / count;
stddev = sqrt((sum_sq / count) - (mean * mean));
}
// 计算图像的直方图
void calc_histogram(unsigned char* data, int width, int height, int* hist) {
int count = width * height;
for (int i = 0; i < count; ++i) {
hist[data[i]]++;
}
}
// 显示直方图
void show_histogram(int* hist) {
int max_count = 0;
for (int i = 0; i <= MAX_VALUE; ++i) {
if (hist[i] > max_count) {
max_count = hist[i];
}
}
for (int i = 0; i <= MAX_VALUE; ++i) {
int count = hist[i];
int height = (int)(((double)count / max_count) * 50); // 调整显示高度
std::cout << i << ": ";
for (int j = 0; j < height; ++j) {
std::cout << "*";
}
std::cout << std::endl;
}
}
int main() {
unsigned char data[] = {
10, 20, 30, 40, 50,
60, 70, 80, 90, 100,
110, 120, 130, 140, 150,
160, 170, 180, 190, 200,
210, 220, 230, 240, 250
};
int width = 5;
int height = 5;
double mean, stddev;
calc_mean_and_stddev(data, width, height, mean, stddev);
std::cout << "Mean: " << mean << std::endl;
std::cout << "Standard Deviation: " << stddev << std::endl;
int hist[MAX_VALUE + 1] = {0};
calc_histogram(data, width, height, hist);
show_histogram(hist);
return 0;
}
```
以上代码演示了如何计算图像的均值和方差,以及如何计算和显示图像的直方图。由于没有使用OpenCV等库函数,因此需要手动读取图像数据并进行处理。在这个例子中,我们使用了一个5x5的灰度图像来演示代码的功能。您可以根据需要修改图像大小和值,以及调整直方图的显示高度等参数。希望这个例子能够帮助您实现自己的代码!
阅读全文
相关推荐
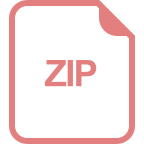
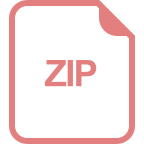
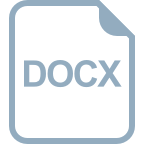
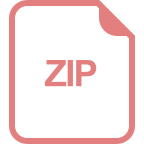
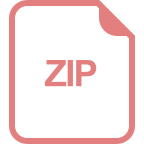
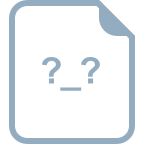
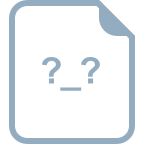