用Java写一段交易系统
时间: 2024-03-03 15:51:30 浏览: 113
好的,以下是一个简单的交易系统的 Java 代码示例:
```java
import java.util.ArrayList;
import java.util.List;
public class TradingSystem {
private List<Double> prices;
private List<Double> ma5;
private List<Double> ma10;
private List<Integer> signals;
private List<Integer> positions;
private List<Double> returns;
public TradingSystem(List<Double> prices) {
this.prices = prices;
this.ma5 = new ArrayList<>();
this.ma10 = new ArrayList<>();
this.signals = new ArrayList<>();
this.positions = new ArrayList<>();
this.returns = new ArrayList<>();
}
public void processData() {
for (int i = 0; i < prices.size(); i++) {
double price = prices.get(i);
if (i < 5) {
ma5.add(price);
} else {
double ma = getMa(prices.subList(i-5, i));
ma5.add(ma);
}
if (i < 10) {
ma10.add(price);
} else {
double ma = getMa(prices.subList(i-10, i));
ma10.add(ma);
}
if (i < 1) {
signals.add(0);
} else {
if (ma5.get(i) > ma10.get(i)) {
signals.add(1);
} else {
signals.add(-1);
}
}
if (i < 1) {
positions.add(0);
} else {
positions.add(signals.get(i-1));
}
if (i < 1) {
returns.add(0.0);
} else {
double pctChange = (price - prices.get(i-1)) / prices.get(i-1);
returns.add(pctChange * positions.get(i-1));
}
}
}
public double getMa(List<Double> data) {
double sum = 0.0;
for (double d : data) {
sum += d;
}
return sum / data.size();
}
public double getProfit() {
double cumReturn = 1.0;
for (double r : returns) {
cumReturn *= (1 + r);
}
return cumReturn - 1;
}
public double getAnnualReturn() {
double profit = getProfit();
int tradingDays = prices.size() - 1;
double annualReturn = Math.pow(1 + profit, 252.0 / tradingDays) - 1;
return annualReturn;
}
public static void main(String[] args) {
List<Double> prices = new ArrayList<>();
prices.add(100.0);
prices.add(101.0);
prices.add(102.0);
prices.add(103.0);
prices.add(104.0);
prices.add(105.0);
prices.add(106.0);
prices.add(107.0);
prices.add(108.0);
prices.add(109.0);
prices.add(110.0);
TradingSystem ts = new TradingSystem(prices);
ts.processData();
System.out.println("收益率: " + ts.getProfit());
System.out.println("年化收益率: " + ts.getAnnualReturn());
}
}
```
这个交易系统主要是基于移动平均线的策略,当5日均线上穿10日均线时,买入股票,当5日均线下穿10日均线时,卖出股票。在这个策略的基础上,我们可以进行更多的优化和改进,比如加入止损、调整均线的参数等等。请注意,这个示例仅供参考,实际交易中需要进行更多的风险控制和数据分析。
阅读全文
相关推荐
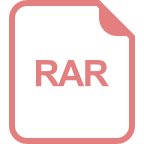
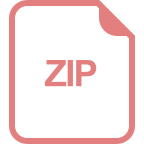
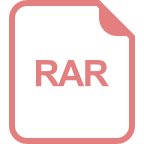
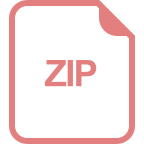
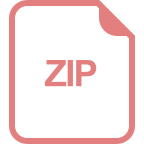
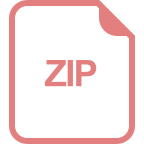
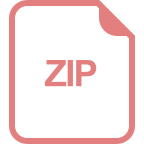
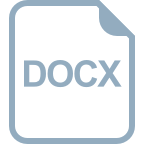
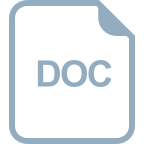
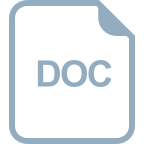
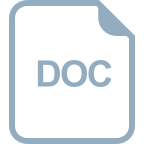
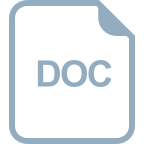
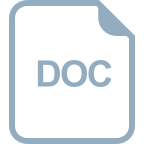
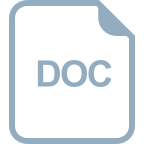
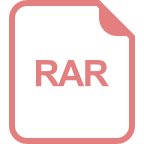