运用vue+springboot实现点赞功能
时间: 2023-09-05 19:13:27 浏览: 285
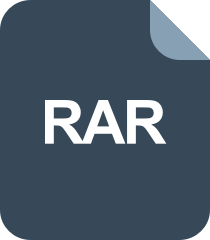
科研项目管理系统,利用springboot+vue实现。

实现点赞功能需要前端和后端的协同工作,具体实现步骤如下:
1. 前端页面实现点赞按钮,点击按钮向后端发送请求。
2. 后端接收到请求后,判断该用户是否已经点过赞,如果没有点过则将点赞记录保存到数据库中,否则返回已经点过赞的提示信息。
3. 前端接收到后端的响应后,根据响应状态进行相应的处理,如更新点赞数、禁用点赞按钮等。
下面是一个简单的实现思路:
1. 前端代码:
```html
<template>
<div>
<button @click="likePost">点赞</button>
<p>{{ likeCount }}人已点赞</p>
</div>
</template>
<script>
export default {
data() {
return {
postId: 1, // 文章ID
likeCount: 0, // 点赞数
isLiked: false // 是否已经点赞
};
},
methods: {
async likePost() {
if (this.isLiked) return; // 如果已经点过赞,则不再发送请求
const res = await this.$axios.post("/like", {
postId: this.postId
});
if (res.data.code === 0) {
this.isLiked = true;
this.likeCount++;
} else {
alert(res.data.msg);
}
}
},
mounted() {
// 获取点赞数和是否已经点赞的状态
this.$axios.get("/like", {
params: {
postId: this.postId
}
}).then(res => {
if (res.data.code === 0) {
this.likeCount = res.data.data.likeCount;
this.isLiked = res.data.data.isLiked;
}
});
}
};
</script>
```
2. 后端代码:
```java
@RestController
public class LikeController {
@Autowired
private LikeService likeService;
@PostMapping("/like")
public Result likePost(@RequestBody Map<String, Object> params) {
Integer postId = (Integer) params.get("postId");
Integer userId = 1; // 假设当前用户ID为1
boolean isLiked = likeService.isLiked(postId, userId);
if (isLiked) {
return Result.fail("您已经点过赞了");
}
likeService.saveLike(postId, userId);
return Result.ok();
}
@GetMapping("/like")
public Result getLikeInfo(Integer postId) {
Integer userId = 1; // 假设当前用户ID为1
LikeStatusDto likeStatus = likeService.getLikeStatus(postId, userId);
return Result.ok(likeStatus);
}
}
@Service
public class LikeServiceImpl implements LikeService {
@Autowired
private LikeMapper likeMapper;
@Override
public boolean isLiked(Integer postId, Integer userId) {
Like like = likeMapper.selectByPostIdAndUserId(postId, userId);
return like != null;
}
@Override
public void saveLike(Integer postId, Integer userId) {
Like like = new Like();
like.setPostId(postId);
like.setUserId(userId);
likeMapper.insert(like);
}
@Override
public LikeStatusDto getLikeStatus(Integer postId, Integer userId) {
int likeCount = likeMapper.countByPostId(postId);
boolean isLiked = isLiked(postId, userId);
LikeStatusDto likeStatus = new LikeStatusDto();
likeStatus.setLikeCount(likeCount);
likeStatus.setLiked(isLiked);
return likeStatus;
}
}
```
其中,`Like`类是一个简单的实体类,包含文章ID和用户ID两个属性;`LikeMapper`是用于操作数据库的Mapper类;`LikeService`是用于处理业务逻辑的Service类;`LikeStatusDto`是用于封装点赞数和是否已经点赞的状态的DTO类。
上述代码中,我们使用了`@PostMapping`和`@GetMapping`注解来处理前端发送的POST和GET请求,同时也使用了SpringBoot提供的注解来注入依赖、处理异常等。
阅读全文
相关推荐
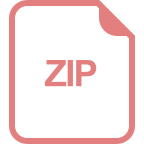
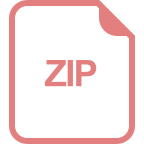
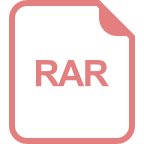
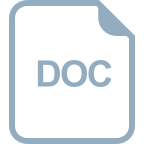
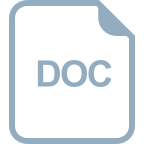
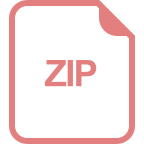
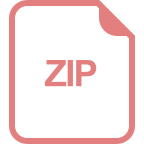
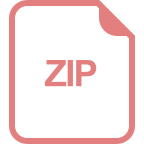
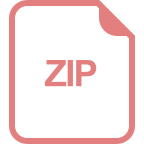
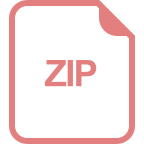
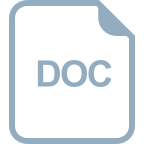
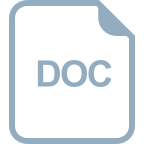
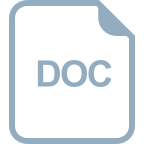
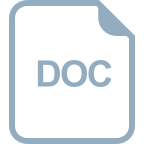
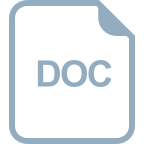
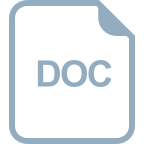