使用c++检查Linux系统硬盘空间,如果空间少于200m时删除最老的文件
时间: 2023-02-24 13:15:14 浏览: 151
下面是一个在C语言中检查Linux系统硬盘空间并删除最旧文件的示例代码:
```
#include <sys/statvfs.h>
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <time.h>
#define THRESHOLD 200000000
int compare(const void *a, const void *b) {
return (*(time_t*)b - *(time_t*)a);
}
int main(void) {
struct statvfs buf;
if (statvfs("/", &buf) == -1) {
perror("statvfs error");
exit(1);
}
unsigned long long free_space = buf.f_bavail * buf.f_frsize;
if (free_space < THRESHOLD) {
DIR *dir;
struct dirent *ent;
dir = opendir("/");
if (dir == NULL) {
perror("opendir error");
exit(1);
}
time_t timestamps[100];
int i = 0;
while ((ent = readdir(dir)) != NULL) {
char path[100];
sprintf(path, "/%s", ent->d_name);
struct stat s;
if (stat(path, &s) == -1) {
perror("stat error");
exit(1);
}
if (!S_ISREG(s.st_mode)) {
continue;
}
timestamps[i++] = s.st_mtime;
}
closedir(dir);
qsort(timestamps, i, sizeof(time_t), compare);
for (int j = 0; j < i; j++) {
char path[100];
sprintf(path, "/%ld", timestamps[j]);
if (remove(path) == -1) {
perror("remove error");
exit(1);
}
free_space += THRESHOLD;
if (free_space >= THRESHOLD) {
break;
}
}
}
return 0;
}
```
请注意,这仅仅是一个示例代码,实际上在生产环境中使用时需要加以修改和完善。
相关推荐
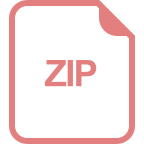
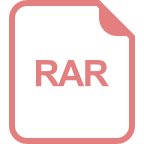
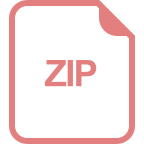














