用python写一个关于ATM取款机项目有PySimpleGUI前段和后端 有注册登录存款取款转账的功能
时间: 2024-03-26 16:36:15 浏览: 192
好的,以下是一个使用 PySimpleGUI 的 Python ATM 取款机项目的示例,包括注册、登录、存款、取款和转账等功能:
前端部分代码:
```python
import PySimpleGUI as sg
# 创建窗口
sg.theme('DarkBlue')
layout = [
[sg.Text('欢迎使用 ATM 取款机')],
[sg.Text('请输入账号'), sg.InputText(key='username')],
[sg.Text('请输入密码'), sg.InputText(key='password', password_char='*')],
[sg.Button('注册'), sg.Button('登录')]
]
window = sg.Window('ATM 取款机', layout, size=(300, 150))
# 进入事件循环
while True:
event, values = window.read()
if event == sg.WIN_CLOSED:
break
elif event == '注册':
# TODO: 注册功能的后端实现
sg.popup('注册成功!')
elif event == '登录':
# TODO: 登录功能的后端实现
sg.popup('登录成功!')
break
# 创建主窗口
layout = [
[sg.Text('欢迎使用 ATM 取款机')],
[sg.Text('账户余额:'), sg.Text('0', key='balance')],
[sg.Button('存款'), sg.Button('取款'), sg.Button('转账'), sg.Button('退出')]
]
window = sg.Window('ATM 取款机', layout)
# 进入事件循环
while True:
event, values = window.read()
if event == sg.WIN_CLOSED or event == '退出':
break
elif event == '存款':
# TODO: 存款功能的后端实现
sg.popup('存款成功!')
elif event == '取款':
# TODO: 取款功能的后端实现
sg.popup('取款成功!')
elif event == '转账':
# TODO: 转账功能的后端实现
sg.popup('转账成功!')
window.close()
```
后端部分代码:
```python
import json
# 加载用户数据
with open('users.json', 'r') as f:
users = json.load(f)
# 注册功能
def register(username, password):
if username in users:
return False
users[username] = {'password': password, 'balance': 0}
with open('users.json', 'w') as f:
json.dump(users, f)
return True
# 登录功能
def login(username, password):
if username not in users:
return False
if users[username]['password'] != password:
return False
return True
# 存款功能
def deposit(username, amount):
if username not in users:
return False
users[username]['balance'] += amount
with open('users.json', 'w') as f:
json.dump(users, f)
return True
# 取款功能
def withdraw(username, amount):
if username not in users:
return False
if users[username]['balance'] < amount:
return False
users[username]['balance'] -= amount
with open('users.json', 'w') as f:
json.dump(users, f)
return True
# 转账功能
def transfer(username, target, amount):
if username not in users or target not in users:
return False
if users[username]['balance'] < amount:
return False
users[username]['balance'] -= amount
users[target]['balance'] += amount
with open('users.json', 'w') as f:
json.dump(users, f)
return True
```
在这个示例中,我们使用 PySimpleGUI 创建了两个窗口,分别用于注册/登录和主操作界面。在注册/登录窗口中,我们通过输入框和按钮实现了注册和登录功能,并在后端使用 JSON 文件存储用户数据。在主操作界面中,我们通过按钮实现了存款、取款和转账等功能,并在后端实现了相应的功能函数。
当用户点击按钮时,前端会调用相应的后端函数进行处理,并通过弹窗提示用户操作结果。注意在实际使用中,需要进一步完善用户输入的验证和异常处理等功能。
阅读全文
相关推荐
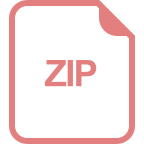
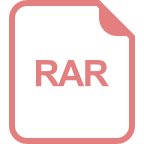
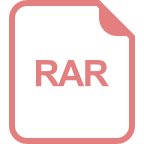
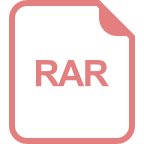
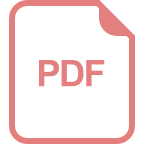
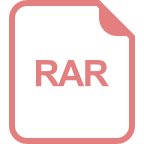
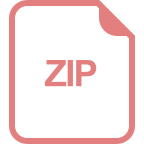
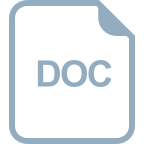
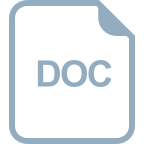
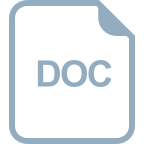
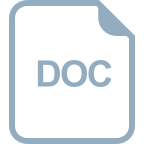



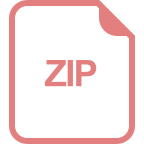
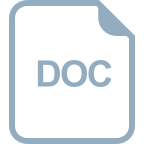

