编写一个c语言程序检测内存泄露
时间: 2023-05-19 13:00:46 浏览: 90
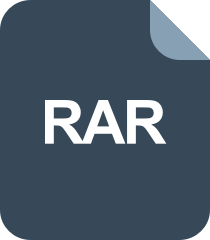
C语言实现的一个内存泄漏检测程序

当涉及到内存泄漏时,我们需要使用一些工具来检测和解决这个问题。其中一个常用的工具是 Valgrind,它可以检测内存泄漏和其它内存错误。以下是一个简单的 C 语言程序,使用 Valgrind 来检测内存泄漏:
```
#include <stdlib.h>
int main() {
int *ptr = malloc(sizeof(int));
*ptr = 42;
free(ptr);
return 0;
}
```
在终端中运行以下命令:
```
valgrind --leak-check=full ./a.out
```
Valgrind 将会输出内存泄漏的详细信息,如果没有内存泄漏,它将会输出类似于以下的信息:
```
==12345== HEAP SUMMARY:
==12345== in use at exit: 0 bytes in 0 blocks
==12345== total heap usage: 1 allocs, 1 frees, 4 bytes allocated
==12345==
==12345== All heap blocks were freed -- no leaks are possible
==12345==
==12345== For counts of detected and suppressed errors, rerun with: -v
==12345== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
```
如果有内存泄漏,它将会输出类似于以下的信息:
```
==12345== HEAP SUMMARY:
==12345== in use at exit: 4 bytes in 1 blocks
==12345== total heap usage: 2 allocs, 1 frees, 8 bytes allocated
==12345==
==12345== 4 bytes in 1 blocks are definitely lost in loss record 1 of 1
==12345== at 0x4C2FB0F: malloc (in /usr/lib/valgrind/vgpreload_memcheck-amd64-linux.so)
==12345== by 0x4005B7: main (in /path/to/program)
==12345==
==12345== LEAK SUMMARY:
==12345== definitely lost: 4 bytes in 1 blocks
==12345== indirectly lost: 0 bytes in 0 blocks
==12345== possibly lost: 0 bytes in 0 blocks
==12345== still reachable: 0 bytes in 0 blocks
==12345== suppressed: 0 bytes in 0 blocks
==12345==
==12345== For counts of detected and suppressed errors, rerun with: -v
==12345== ERROR SUMMARY: 1 errors from 1 contexts (suppressed: 0 from 0)
```
这个程序中没有内存泄漏,所以 Valgrind 输出了 "no leaks are possible" 的信息。
阅读全文
相关推荐
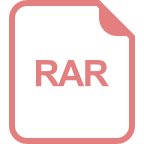
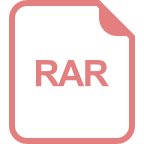
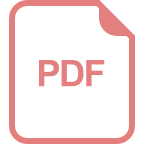
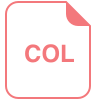

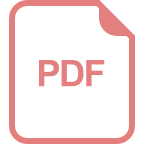
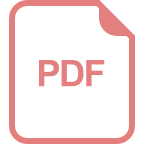
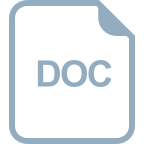
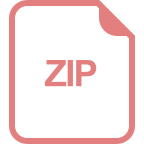
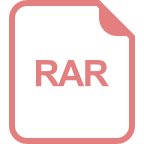
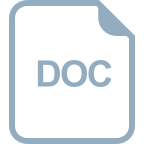
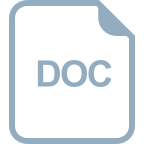
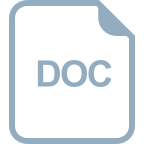
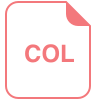
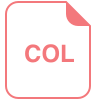

