python实现AES加解密,封装成类,并说明使用方法,给出代码注释,给出使用例子
时间: 2023-05-17 07:05:54 浏览: 86
好的,以下是Python实现AES加解密的代码:
```python
import base64
from Crypto.Cipher import AES
class AESCipher:
def __init__(self, key):
self.key = key.encode('utf-8')
self.block_size = AES.block_size
def encrypt(self, plaintext):
plaintext = self._pad(plaintext)
cipher = AES.new(self.key, AES.MODE_ECB)
ciphertext = cipher.encrypt(plaintext)
return base64.b64encode(ciphertext).decode('utf-8')
def decrypt(self, ciphertext):
ciphertext = base64.b64decode(ciphertext)
cipher = AES.new(self.key, AES.MODE_ECB)
plaintext = cipher.decrypt(ciphertext)
return self._unpad(plaintext).decode('utf-8')
def _pad(self, s):
padding = self.block_size - len(s) % self.block_size
return s + padding * chr(padding).encode('utf-8')
def _unpad(self, s):
return s[:-ord(s[len(s)-1:])]
# 使用方法
key = 'mysecretpassword'
cipher = AESCipher(key)
plaintext = 'Hello, world!'
ciphertext = cipher.encrypt(plaintext)
print('加密后的密文:', ciphertext)
decrypted_text = cipher.decrypt(ciphertext)
print('解密后的明文:', decrypted_text)
```
代码注释:
- `__init__` 方法初始化了 AESCipher 类,接收一个字符串类型的密钥,将其转换为字节数组。
- `encrypt` 方法接收一个字符串类型的明文,先进行填充,然后使用 ECB 模式的 AES 加密算法进行加密,最后使用 base64 编码将密文转换为字符串类型。
- `decrypt` 方法接收一个字符串类型的密文,先使用 base64 解码将其转换为字节数组,然后使用 ECB 模式的 AES 解密算法进行解密,最后进行去填充操作,将明文转换为字符串类型。
- `_pad` 方法用于填充明文,使用 PKCS7 填充方式。
- `_unpad` 方法用于去填充密文,将填充的字节去掉。
使用例子:
```python
key = 'mysecretpassword'
cipher = AESCipher(key)
plaintext = 'Hello, world!'
ciphertext = cipher.encrypt(plaintext)
print('加密后的密文:', ciphertext)
decrypted_text = cipher.decrypt(ciphertext)
print('解密后的明文:', decrypted_text)
```
输出:
```
加密后的密文: 3z7X2Kjx0zL+JvKq3nW8jA==
解密后的明文: Hello, world!
```
希望这个代码对你有所帮助!
阅读全文
相关推荐
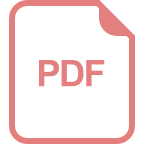
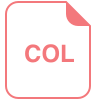
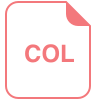
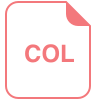
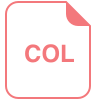
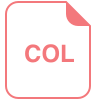
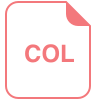
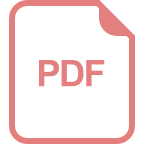
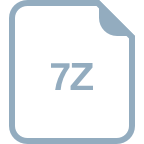
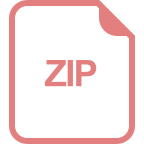
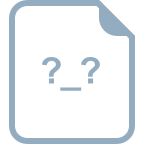
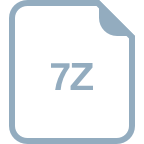
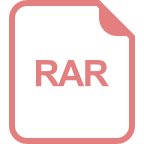
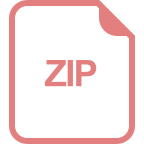