提供几个Jackson Mixin的功能示例
时间: 2023-04-06 07:01:13 浏览: 238
好的,以下是几个 Jackson Mixin 的功能示例:
1. 为某个类添加序列化和反序列化规则:
```
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "type")
@JsonSubTypes({
@JsonSubTypes.Type(value = Dog.class, name = "dog"),
@JsonSubTypes.Type(value = Cat.class, name = "cat")
})
public abstract class AnimalMixin {
@JsonCreator
public AnimalMixin(@JsonProperty("name") String name) {}
@JsonProperty("name")
abstract String getName();
}
```
2. 为某个属性添加序列化和反序列化规则:
```
public class Person {
private String name;
private int age;
// getters and setters
}
public interface PersonMixin {
@JsonProperty("fullName")
String getName();
@JsonIgnore
int getAge();
}
ObjectMapper mapper = new ObjectMapper();
mapper.addMixIn(Person.class, PersonMixin.class);
```
3. 为某个类添加自定义序列化和反序列化规则:
```
public class User {
private String username;
private String password;
// getters and setters
}
public abstract class UserMixin {
@JsonCreator
public UserMixin(@JsonProperty("username") String username, @JsonProperty("password") String password) {}
@JsonProperty("username")
abstract String getUsername();
@JsonProperty("password")
abstract String getPassword();
}
public class UserDeserializer extends StdDeserializer<User> {
public UserDeserializer() {
super(User.class);
}
@Override
public User deserialize(JsonParser p, DeserializationContext ctxt) throws IOException, JsonProcessingException {
JsonNode node = p.getCodec().readTree(p);
String username = node.get("username").asText();
String password = node.get("password").asText();
return new User(username.toUpperCase(), password);
}
}
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.setMixInAnnotation(User.class, UserMixin.class);
module.addDeserializer(User.class, new UserDeserializer());
mapper.registerModule(module);
```
阅读全文
相关推荐
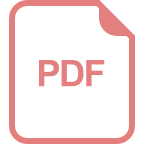
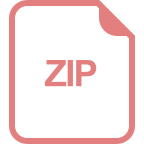
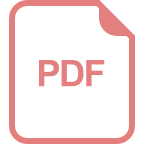
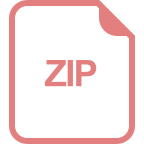
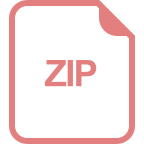
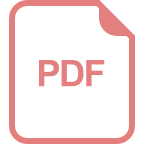
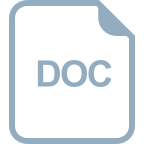
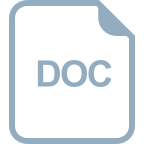
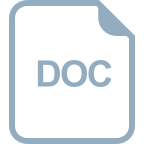
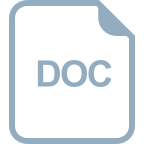
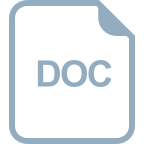




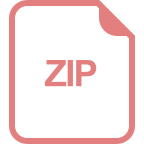