基于java和sqlserver的图书管理系统
时间: 2023-10-10 19:14:01 浏览: 275
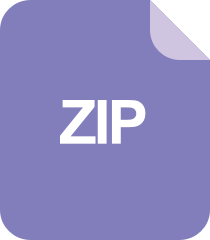
课程设计-图书管理系统(Java+SQLServer)
好的,下面是一个基于 Java 和 SQL Server 数据库的图书管理系统的代码示例:
首先,需要在 SQL Server 数据库中创建一个名为 `library` 的数据库,并在其中创建一个名为 `books` 的表,包括以下字段:
| Field | Type | Null | Key | Default | Extra |
| --------- | ------------ | ---- | ---- | ------- | -------------- |
| id | int | NO | PRI | NULL | identity(1,1) |
| title | nvarchar(50) | NO | | NULL | |
| author | nvarchar(50) | NO | | NULL | |
| available | bit | NO | | 1 | |
| price | money | NO | | NULL | |
接下来,可以使用 JDBC API 连接到数据库,并执行 SQL 语句进行数据的插入、删除和查询操作。以下是一个示例代码:
```java
import java.math.BigDecimal;
import java.sql.*;
import java.util.ArrayList;
import java.util.Scanner;
class Book {
private int id;
private String title;
private String author;
private boolean available;
private BigDecimal price;
public Book(int id, String title, String author, boolean available, BigDecimal price) {
this.id = id;
this.title = title;
this.author = author;
this.available = available;
this.price = price;
}
public int getId() {
return id;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public boolean isAvailable() {
return available;
}
public void setAvailable(boolean available) {
this.available = available;
}
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
}
class Library {
private ArrayList<Book> books;
public Library(ArrayList<Book> books) {
this.books = books;
}
public ArrayList<Book> getBooks() {
return books;
}
public void setBooks(ArrayList<Book> books) {
this.books = books;
}
public void addBook(Book book, Connection conn) throws SQLException {
PreparedStatement stmt = conn.prepareStatement("INSERT INTO books (title, author, available, price) VALUES (?, ?, ?, ?)");
stmt.setString(1, book.getTitle());
stmt.setString(2, book.getAuthor());
stmt.setBoolean(3, book.isAvailable());
stmt.setBigDecimal(4, book.getPrice());
stmt.executeUpdate();
stmt.close();
}
public void removeBook(int id, Connection conn) throws SQLException {
PreparedStatement stmt = conn.prepareStatement("DELETE FROM books WHERE id = ?");
stmt.setInt(1, id);
stmt.executeUpdate();
stmt.close();
}
public ArrayList<Book> getAllBooks(Connection conn) throws SQLException {
ArrayList<Book> books = new ArrayList<>();
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM books");
while (rs.next()) {
int id = rs.getInt("id");
String title = rs.getString("title");
String author = rs.getString("author");
boolean available = rs.getBoolean("available");
BigDecimal price = rs.getBigDecimal("price");
books.add(new Book(id, title, author, available, price));
}
rs.close();
stmt.close();
return books;
}
public void displayBooks() {
System.out.println("ID\tTitle\tAuthor\tAvailable\tPrice");
for (Book book : books) {
System.out.println(book.getId() + "\t" + book.getTitle() + "\t" + book.getAuthor() + "\t" + book.isAvailable() + "\t" + book.getPrice());
}
}
}
public class BookManagementSystem {
public static void main(String[] args) {
String url = "jdbc:sqlserver://localhost:1433;databaseName=library";
String user = "sa";
String password = "password";
ArrayList<Book> books = new ArrayList<>();
try {
Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement stmt = conn.prepareStatement("CREATE TABLE IF NOT EXISTS books (id INT NOT NULL IDENTITY(1,1), title NVARCHAR(50) NOT NULL, author NVARCHAR(50) NOT NULL, available BIT NOT NULL DEFAULT 1, price MONEY NOT NULL, PRIMARY KEY (id))");
stmt.executeUpdate();
stmt.close();
Library library = new Library(books);
Scanner scanner = new Scanner(System.in);
int choice;
do {
System.out.println("1. Add book");
System.out.println("2. Remove book");
System.out.println("3. Display books");
System.out.println("0. Exit");
System.out.print("Enter your choice: ");
choice = scanner.nextInt();
switch (choice) {
case 1:
scanner.nextLine(); // Consume newline character
System.out.print("Enter book title: ");
String title = scanner.nextLine();
System.out.print("Enter book author: ");
String author = scanner.nextLine();
System.out.print("Enter book price: ");
BigDecimal price = scanner.nextBigDecimal();
library.addBook(new Book(0, title, author, true, price), conn);
break;
case
阅读全文
相关推荐
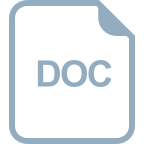
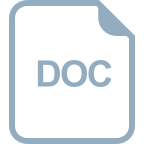
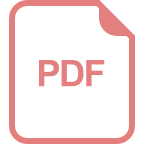
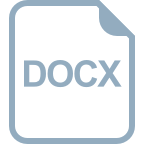
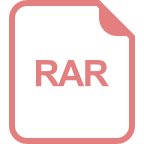
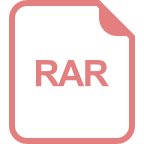
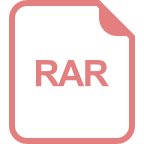
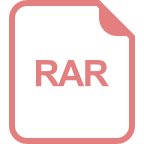
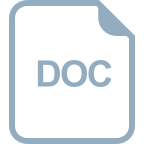
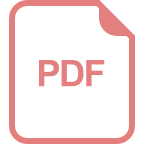
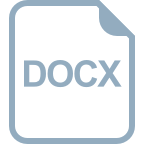
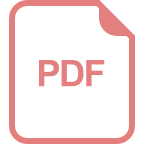
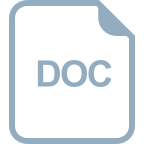

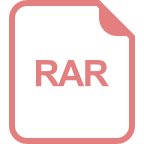
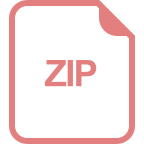
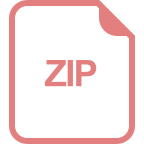