qtextedit 显示行号
时间: 2023-09-26 07:06:04 浏览: 69
要在 QTextEdit 中显示行号,您需要将 QTextEdit 放入 QWidget 中,并使用 QVBoxLayout 将 QTextEdit 和 QPlainTextEdit(用于显示行号)垂直地放置在一起。然后,您可以使用 QPlainTextEdit 的 setPlainText() 方法将文本复制到 QPlainTextEdit 中,并使用 QPlainTextEdit 的 lineNumberAreaPaintEvent() 方法绘制行号。
以下是一个简单的示例:
```python
from PyQt5.QtCore import Qt, QRect, QSize
from PyQt5.QtGui import QTextCursor, QPainter, QTextFormat, QColor
from PyQt5.QtWidgets import QWidget, QTextEdit, QVBoxLayout, QPlainTextEdit
class LineNumberArea(QWidget):
def __init__(self, editor):
super().__init__(editor)
self.editor = editor
def sizeHint(self):
return QSize(self.editor.lineNumberAreaWidth(), 0)
def paintEvent(self, event):
self.editor.lineNumberAreaPaintEvent(event)
class TextEditor(QTextEdit):
def __init__(self):
super().__init__()
self.lineNumberArea = LineNumberArea(self)
layout = QVBoxLayout()
layout.addWidget(self.lineNumberArea)
layout.addWidget(self)
widget = QWidget()
widget.setLayout(layout)
self.setAcceptRichText(False)
self.setVerticalScrollBarPolicy(Qt.ScrollBarAlwaysOn)
self.setHorizontalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setLineWrapMode(QTextEdit.NoWrap)
self.blockCountChanged.connect(self.updateLineNumberAreaWidth)
self.updateRequest.connect(self.updateLineNumberArea)
self.cursorPositionChanged.connect(self.highlightCurrentLine)
self.updateLineNumberAreaWidth(0)
def lineNumberAreaWidth(self):
digits = 1
count = max(1, self.blockCount())
while count >= 10:
count /= 10
digits += 1
space = 3 + self.fontMetrics().width('9') * digits
return space
def updateLineNumberAreaWidth(self, _):
self.setViewportMargins(self.lineNumberAreaWidth(), 0, 0, 0)
def updateLineNumberArea(self, rect, dy):
if dy:
self.lineNumberArea.scroll(0, dy)
else:
self.lineNumberArea.update(0, rect.y(), self.lineNumberArea.width(),
rect.height())
if rect.contains(self.viewport().rect()):
self.updateLineNumberAreaWidth(0)
def resizeEvent(self, event):
super().resizeEvent(event)
cr = self.contentsRect()
self.lineNumberArea.setGeometry(QRect(cr.left(), cr.top(),
self.lineNumberAreaWidth(), cr.height()))
def lineNumberAreaPaintEvent(self, event):
painter = QPainter(self.lineNumberArea)
painter.fillRect(event.rect(), QColor(240, 240, 240))
block = self.firstVisibleBlock()
blockNumber = block.blockNumber()
top = self.blockBoundingGeometry(block).translated(self.contentOffset()).top()
bottom = top + self.blockBoundingRect(block).height()
height = self.fontMetrics().height()
while block.isValid() and (top <= event.rect().bottom()):
if block.isVisible() and (bottom >= event.rect().top()):
number = str(blockNumber + 1)
painter.setPen(Qt.black)
painter.drawText(0, top, self.lineNumberArea.width(), height,
Qt.AlignRight, number)
block = block.next()
top = bottom
bottom = top + self.blockBoundingRect(block).height()
blockNumber += 1
def highlightCurrentLine(self):
extraSelections = []
if not self.isReadOnly():
selection = QTextEdit.ExtraSelection()
lineColor = QColor(Qt.yellow).lighter(160)
selection.format.setBackground(lineColor)
selection.format.setProperty(QTextFormat.FullWidthSelection, True)
selection.cursor = self.textCursor()
selection.cursor.clearSelection()
extraSelections.append(selection)
self.setExtraSelections(extraSelections)
```
您可以使用以下代码创建 TextEditor:
```python
text_editor = TextEditor()
```
然后,您可以将文本添加到 QTextEdit 中,并将其设置为 QPlainTextEdit:
```python
text = 'Hello world!'
text_editor.setPlainText(text)
```
现在,您可以在行号区域中看到行号,如下所示:

相关推荐










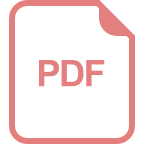