给tableview设置带变量的文本
时间: 2024-02-15 20:03:13 浏览: 68
你可以使用`attributedText`属性来设置带变量的文本。首先,定义一个字符串,其中包含变量占位符,例如:
```
let text = "Hello, {name}! Your score is {score}."
```
然后,使用`NSMutableAttributedString`类创建一个带有属性的字符串,并将变量替换为实际值:
```
let attributedText = NSMutableAttributedString(string: text)
let name = "John"
let score = 100
let nameRange = (text as NSString).range(of: "{name}")
let scoreRange = (text as NSString).range(of: "{score}")
attributedText.replaceCharacters(in: nameRange, with: name)
attributedText.replaceCharacters(in: scoreRange, with: "\(score)")
```
最后,将带有属性的字符串设置为`tableview`的`attributedText`属性即可:
```
cell.textLabel?.attributedText = attributedText
```
相关问题
qt中tableview复制粘贴
在Qt中,可以使用QTableView类来创建一个表格视图。如果你想在表格视图中实现复制粘贴功能,可以通过以下步骤来实现:
1. 在你的QTableView类中,添加以下代码:
```
QShortcut *copyShortcut = new QShortcut(QKeySequence::Copy, this);
connect(copyShortcut, &QShortcut::activated, this, &MyTableView::copy);
QShortcut *pasteShortcut = new QShortcut(QKeySequence::Paste, this);
connect(pasteShortcut, &QShortcut::activated, this, &MyTableView::paste);
```
这里使用了QShortcut类来创建复制和粘贴的快捷键,并将其连接到copy()和paste()槽函数。
2. 实现copy()和paste()槽函数:
```
void MyTableView::copy()
{
QModelIndexList selectedIndexes = selectionModel()->selectedIndexes();
if (selectedIndexes.isEmpty())
return;
qSort(selectedIndexes);
QString text;
int currentRow = selectedIndexes.first().row();
foreach (const QModelIndex &index, selectedIndexes) {
if (index.row() != currentRow) {
text += '\n';
currentRow = index.row();
} else if (text.length() > 0) {
text += '\t';
}
text += index.data(Qt::DisplayRole).toString();
}
QClipboard *clipboard = QApplication::clipboard();
clipboard->setText(text);
}
void MyTableView::paste()
{
QClipboard *clipboard = QApplication::clipboard();
QString text = clipboard->text();
QModelIndexList selectedIndexes = selectionModel()->selectedIndexes();
if (selectedIndexes.isEmpty())
return;
qSort(selectedIndexes);
int row = selectedIndexes.first().row();
int col = selectedIndexes.first().column();
QStringList lines = text.split('\n');
foreach (const QString &line, lines) {
QStringList fields = line.split('\t');
foreach (const QString &field, fields) {
QModelIndex index = model()->index(row, col);
model()->setData(index, field, Qt::EditRole);
col++;
}
row++;
col = selectedIndexes.first().column();
}
}
```
在copy()函数中,我们首先获取当前选中的单元格的索引,然后按行列顺序遍历所有选中的单元格,并将其内容复制到一个QString变量中。最后,将复制的文本设置到剪贴板上。
在paste()函数中,我们首先从剪贴板获取文本,并将其按行列分割成单元格内容。然后,遍历选中的单元格,将剪贴板中的文本依次粘贴到每个单元格中。
3. 最后,在你的QTableView类中添加以下头文件:
```
#include <QShortcut>
#include <QApplication>
#include <QClipboard>
```
这些头文件包含了我们需要使用的类和函数。
void user_manage::on_btn_user_search_clicked() { // 读取文件 QFile file("users.txt"); if (!file.open(QIODevice::ReadOnly | QIODevice::Text)) { return; } // 创建一个数据模型 QStandardItemModel* model = new QStandardItemModel(this); model->setColumnCount(2); // 设置表头 model->setHeaderData(0, Qt::Horizontal, "用户名"); model->setHeaderData(1, Qt::Horizontal, "密码"); // 获取搜索关键字 QString keyword = ui->user_searchEdit->text().trimmed(); // 按行读取文件并搜索匹配的行 QTextStream in(&file); in.setCodec("UTF-8"); while(!in.atEnd()) { QString line = in.readLine(); // 分割每一行的文本 QStringList parts = line.split(",", QString::KeepEmptyParts); // 检查用户名是否与关键字匹配 QString username = parts.value(0); if (username.contains(keyword, Qt::CaseInsensitive)) { // 创建一行并添加到模型中 QList<QStandardItem*> rowItems; for (const QString& part : parts) { QStandardItem* item = new QStandardItem(part); rowItems.append(item); } model->appendRow(rowItems); } } file.close(); // 显示用户数据 ui->tableView->setModel(model); } 画个流程图
抱歉,我无法直接画流程图。但是,我可以为您提供一个伪代码示例,帮助您理解该函数的执行流程:
```plaintext
1. 点击搜索按钮(on_btn_user_search_clicked)触发该函数。
2. 打开名为"users.txt"的文件。
3. 如果文件打开失败,则退出函数。
4. 创建一个名为model的QStandardItemModel数据模型,并设置列数为2。
5. 设置表头为"用户名"和"密码"。
6. 从用户界面获取搜索关键字。
7. 使用UTF-8编码创建一个文本流in,读取文件内容。
8. 循环遍历文件中的每一行,直到文件末尾。
9. 读取一行文本并将其存储在变量line中。
10. 使用逗号分割每一行的文本,得到一个QStringList parts。
11. 检查用户名(parts的第一个值)是否与关键字匹配(不区分大小写)。
12. 如果匹配,则创建一个QList<QStandardItem*>类型的rowItems列表。
13. 遍历parts中的每个值,创建一个QStandardItem对象,并将其添加到rowItems列表中。
14. 将rowItems列表添加到model中作为一行数据。
15. 关闭文件。
16. 将model设置为用户界面的tableView视图的模型,以显示用户数据。
```
希望这个伪代码示例可以帮助您理解该函数的执行流程。如果您有任何进一步的问题,请随时提问!
阅读全文
相关推荐
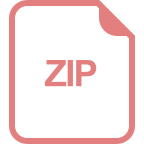
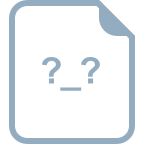
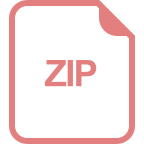
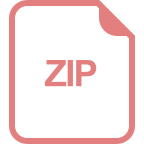
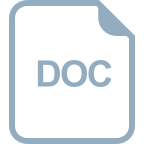




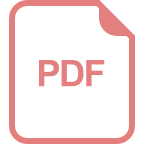
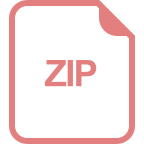
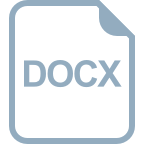
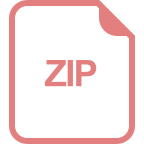