TypeError: a bytes-like object is required, not '_io.BufferedReader'
时间: 2023-08-10 15:13:57 浏览: 788
这个错误通常是因为您正在尝试将一个文件对象传递给一个期望字符串或字节的函数。
您可以使用 `.read()` 方法将文件对象转换为字符串或字节,然后再传递给函数。
例如:
```python
with open('file.txt', 'rb') as f:
data = f.read()
some_function(data) # 期望字节类型的函数
```
或者,如果您期望的是字符串类型的函数,则可以使用 `.decode()` 方法将字节转换为字符串:
```python
with open('file.txt', 'rb') as f:
data = f.read()
data_str = data.decode('utf-8') # 将字节解码为字符串
some_function(data_str) # 期望字符串类型的函数
```
请注意,您需要根据您的具体情况选择正确的编码(例如,如果您的文件使用的是 UTF-8 编码,则需要将其解码为 UTF-8)。
相关问题
TypeError: a bytes-like object is required, not '_io.BytesIO'
如果您遇到了`TypeError: a bytes-like object is required, not '_io.BytesIO'`错误,这通常表示您需要将`BytesIO`对象转换为字节串对象。
您可以使用`BytesIO.getvalue()`方法将`BytesIO`对象中的数据转换为字节串对象,例如:
```python
import io
# 创建一个新的BytesIO对象
file_attributes = io.BytesIO()
# 向BytesIO对象中写入数据
file_attributes.write(b'Hello, world!')
# 将BytesIO对象转换为字节串对象
file_attributes_bytes = file_attributes.getvalue()
# 关闭BytesIO对象
file_attributes.close()
# 打印字节串对象
print(file_attributes_bytes)
```
输出:
```
b'Hello, world!'
```
在上面的示例中,我们创建了一个新的`BytesIO`对象,并向其中写入了一个字节串`b'Hello, world!'`。接着,我们使用`BytesIO.getvalue()`方法将`BytesIO`对象中的数据转换为字节串对象,并将其存储在`file_attributes_bytes`变量中。最后,我们关闭`BytesIO`对象并打印字节串对象。
请注意,如果您想继续使用`BytesIO`对象,而不是将其转换为字节串对象,请确保在使用`BytesIO`对象时传递的参数是字节串对象。
TypeError: a bytes-like object is required, not 'int'
当出现TypeError: a bytes-like object is required, not 'int'错误时,这通常是因为在socket中传递的数据类型不正确。socket只能传递bytes类型的数据,而不能传递int类型的数据。要解决这个问题,你需要将int类型的数据转换为bytes类型。
一种解决方法是使用to_bytes()方法将int类型的数据转换为bytes类型。例如,可以使用以下代码将一个整数转换为6字节的大字节序的bytes类型数据:
```python
x = 10
connect.send(x.to_bytes(length=6, byteorder='big'))
```
这将把整数10转换为一个6字节的bytes类型数据,并将其发送到socket中。
另一种解决方法是使用str()函数将int类型的数据转换为字符串,然后再使用encode()方法将字符串转换为bytes类型。例如:
```python
x = 10
connect.send(str(x).encode())
```
这将把整数10转换为一个字符串"10",然后再将其转换为bytes类型数据,并发送到socket中。
综上所述,当出现TypeError: a bytes-like object is required, not 'int'错误时,你可以使用to_bytes()方法或者str()函数和encode()方法将int类型的数据转换为bytes类型,从而解决这个问题。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [关于socket执行send()语句时,例如报错TypeError: a bytes-like object is required, not ‘list’等,...](https://blog.csdn.net/zfanfan/article/details/127411683)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
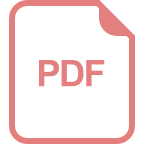
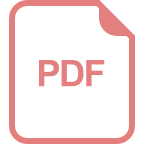
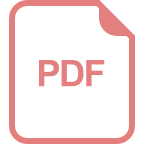












