asp .net. MVC 增删改查
时间: 2024-04-30 21:24:26 浏览: 178
ASP.NET MVC 是一种基于模型-视图-控制器 (MVC) 架构的 Web 应用程序框架。它提供了一种创建 Web 应用程序的模式,可以使开发人员将应用程序的逻辑、布局和用户界面分开。
在 ASP.NET MVC 中,常见的增删改查操作可以使用以下步骤完成:
1. 创建模型类:定义要操作的实体类。
2. 创建控制器类:定义处理请求和响应的代码,包括数据操作和视图渲染。
3. 创建视图:定义呈现数据的 HTML 界面。
4. 实现数据操作:可以使用 Entity Framework 或者其他 ORM 框架来实现数据库的增删改查操作。
下面是一个简单的示例,演示如何在 ASP.NET MVC 中实现增删改查操作:
1. 创建模型类:
```
public class Book
{
public int BookId { get; set; }
public string Title { get; set; }
public string Author { get; set; }
public decimal Price { get; set; }
}
```
2. 创建控制器类:
```
public class BookController : Controller
{
// 显示书籍列表
public ActionResult Index()
{
var books = db.Books.ToList();
return View(books);
}
// 添加书籍
public ActionResult Add()
{
return View();
}
[HttpPost]
public ActionResult Add(Book book)
{
db.Books.Add(book);
db.SaveChanges();
return RedirectToAction("Index");
}
// 修改书籍
public ActionResult Edit(int id)
{
var book = db.Books.Find(id);
return View(book);
}
[HttpPost]
public ActionResult Edit(Book book)
{
db.Entry(book).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
// 删除书籍
public ActionResult Delete(int id)
{
var book = db.Books.Find(id);
db.Books.Remove(book);
db.SaveChanges();
return RedirectToAction("Index");
}
private ApplicationDbContext db = new ApplicationDbContext();
}
```
3. 创建视图:
```
@model IEnumerable<Book>
<h2>书籍列表</h2>
<p>
@Html.ActionLink("添加书籍", "Add")
</p>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.Title)
</th>
<th>
@Html.DisplayNameFor(model => model.Author)
</th>
<th>
@Html.DisplayNameFor(model => model.Price)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Title)
</td>
<td>
@Html.DisplayFor(modelItem => item.Author)
</td>
<td>
@Html.DisplayFor(modelItem => item.Price)
</td>
<td>
@Html.ActionLink("编辑", "Edit", new { id=item.BookId }) |
@Html.ActionLink("删除", "Delete", new { id=item.BookId })
</td>
</tr>
}
</table>
```
```
@model Book
<h2>添加书籍</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-group">
@Html.LabelFor(model => model.Title, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Title, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Title, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Author, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Author, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Author, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="添加" class="btn btn-default" />
</div>
</div>
}
<div>
@Html.ActionLink("返回列表", "Index")
</div>
```
```
@model Book
<h2>编辑书籍</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-group">
@Html.LabelFor(model => model.Title, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Title, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Title, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Author, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Author, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Author, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="保存" class="btn btn-default" />
</div>
</div>
}
<div>
@Html.ActionLink("返回列表", "Index")
</div>
```
4. 实现数据操作:
可以使用 Entity Framework 来实现数据库的增删改查操作,具体代码可以参考以下示例:
```
public class ApplicationDbContext : DbContext
{
public DbSet<Book> Books { get; set; }
}
```
注意,在使用 Entity Framework 之前,还需要进行一些配置,例如在 Web.config 中添加连接字符串。
阅读全文
相关推荐














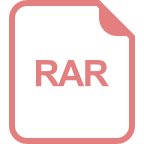



