用python编写网页版的聊天机器人
时间: 2023-07-27 19:16:44 浏览: 86
你可以使用Python的Flask框架来编写网页版的聊天机器人。以下是一个简单的示例代码:
1. 安装Flask框架和ChatterBot库:
```python
pip install flask
pip install chatterbot
```
2. 创建Flask应用程序并定义一个简单的路由:
```python
from flask import Flask, render_template, request
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
app = Flask(__name__)
# Create a chatbot
bot = ChatBot('MyBot')
trainer = ChatterBotCorpusTrainer(bot)
trainer.train("chatterbot.corpus.english")
# Define a route for the homepage
@app.route('/')
def index():
return render_template('index.html')
# Define a route for the chatbot
@app.route('/chatbot', methods=['POST'])
def chatbot():
user_input = request.form['user_input']
bot_response = str(bot.get_response(user_input))
return bot_response
if __name__ == '__main__':
app.run(debug=True)
```
3. 创建一个HTML模板来呈现聊天界面:
```html
<!doctype html>
<html>
<head>
<title>Chatbot</title>
</head>
<body>
<h1>Chatbot</h1>
<div id="chat-log"></div>
<form>
<input type="text" id="user-input" placeholder="Say something...">
<button type="submit">Send</button>
</form>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(function() {
$('form').submit(function(event) {
event.preventDefault();
var user_input = $('#user-input').val();
$('#chat-log').append('<p><strong>You:</strong> ' + user_input + '</p>');
$.post('/chatbot', {'user_input': user_input}, function(data) {
$('#chat-log').append('<p><strong>Bot:</strong> ' + data + '</p>');
});
$('#user-input').val('');
});
});
</script>
</body>
</html>
```
4. 运行应用程序并在浏览器中访问http://localhost:5000/,即可开始与聊天机器人交互。
以上代码仅是一个简单的示例,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
















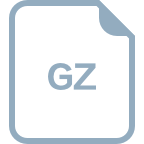
