python设计界面,选择读取文件,设置密钥,对不同类型文件进行加密,输出文件位置
时间: 2023-12-31 18:04:24 浏览: 74
要实现这样的界面,你可以使用Python的GUI库,比如Tkinter或者PyQt。下面是一个简单的示例代码,演示了如何使用Tkinter实现一个简单的加密工具界面:
```
import tkinter as tk
from tkinter import filedialog
from Crypto.Cipher import AES
class Encryptor:
def __init__(self, key):
self.key = key.encode('utf-8')
self.cipher = AES.new(self.key, AES.MODE_EAX)
def encrypt_file(self, file_in, file_out):
with open(file_in, 'rb') as f_in:
data = f_in.read()
ciphertext, tag = self.cipher.encrypt_and_digest(data)
with open(file_out, 'wb') as f_out:
[f_out.write(x) for x in (self.cipher.nonce, tag, ciphertext)]
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.select_file_button = tk.Button(self, text="Select File", command=self.select_file)
self.select_file_button.pack()
self.key_label = tk.Label(self, text="Key:")
self.key_label.pack()
self.key_entry = tk.Entry(self, show="*")
self.key_entry.pack()
self.encrypt_button = tk.Button(self, text="Encrypt", command=self.encrypt_file)
self.encrypt_button.pack()
def select_file(self):
self.file_in = filedialog.askopenfilename()
def encrypt_file(self):
key = self.key_entry.get()
encryptor = Encryptor(key)
file_out = filedialog.asksaveasfilename()
encryptor.encrypt_file(self.file_in, file_out)
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
在这个代码中,我们定义了一个`Encryptor`类,用于加密文件。在`Application`类中,我们创建了一个包含"Select File"按钮、"Key"标签、"Key"输入框和"Encrypt"按钮的界面。当用户点击"Select File"按钮时,会弹出文件选择对话框,用户可以选择要加密的文件。当用户点击"Encrypt"按钮时,程序会读取用户输入的密钥,将选择的文件进行加密,并将加密后的结果写入用户选择的输出文件中。
注意,这个示例代码只是一个简单的示例,实际上还需要添加一些错误处理代码,比如当用户没有选择加密文件时,应该给出提示。
阅读全文
相关推荐
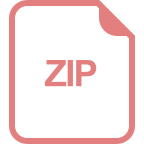
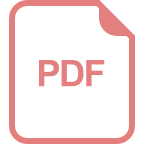

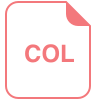














