实现论坛管理,在mysql中创建论坛表,包含留言时间、留言IP地址、留言内容并且在servlet程序中实现发表留言、留言显示、删除留言
时间: 2024-05-05 15:15:10 浏览: 129
1. 创建论坛表
在MySQL中创建论坛表,包括留言时间、留言IP地址、留言内容等字段。
```
CREATE TABLE forum (
id INT(11) NOT NULL AUTO_INCREMENT,
post_time TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
ip VARCHAR(20) NOT NULL,
content TEXT NOT NULL,
PRIMARY KEY (id)
);
```
2. 实现发表留言
在Servlet中实现发表留言功能,将用户输入的留言内容、留言IP地址等信息插入到论坛表中。
```
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String content = request.getParameter("content");
String ip = request.getRemoteAddr();
try {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement pstmt = conn.prepareStatement("INSERT INTO forum (ip, content) VALUES (?, ?)");
pstmt.setString(1, ip);
pstmt.setString(2, content);
pstmt.executeUpdate();
pstmt.close();
conn.close();
response.sendRedirect("index.jsp");
} catch (Exception e) {
e.printStackTrace();
}
}
```
3. 实现留言显示
在Servlet中实现留言显示功能,从论坛表中查询留言记录,并将结果封装成List对象,通过JSP页面展示留言列表。
```
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
Statement stmt = conn.createStatement();
String sql = "SELECT * FROM forum ORDER BY post_time DESC";
ResultSet rs = stmt.executeQuery(sql);
List<ForumPost> posts = new ArrayList<>();
while (rs.next()) {
ForumPost post = new ForumPost();
post.setId(rs.getInt("id"));
post.setPostTime(rs.getTimestamp("post_time"));
post.setIp(rs.getString("ip"));
post.setContent(rs.getString("content"));
posts.add(post);
}
rs.close();
stmt.close();
conn.close();
request.setAttribute("posts", posts);
request.getRequestDispatcher("index.jsp").forward(request, response);
} catch (Exception e) {
e.printStackTrace();
}
}
```
4. 实现删除留言
在Servlet中实现删除留言功能,根据留言ID从论坛表中删除对应记录。
```
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int postId = Integer.parseInt(request.getParameter("postId"));
try {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement pstmt = conn.prepareStatement("DELETE FROM forum WHERE id = ?");
pstmt.setInt(1, postId);
pstmt.executeUpdate();
pstmt.close();
conn.close();
response.sendRedirect("index.jsp");
} catch (Exception e) {
e.printStackTrace();
}
}
```
阅读全文
相关推荐
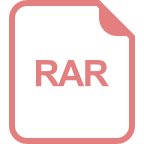
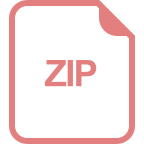


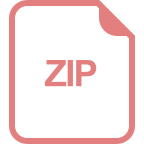
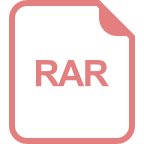
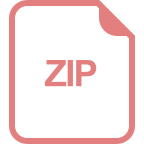
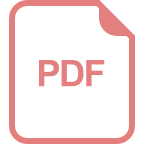
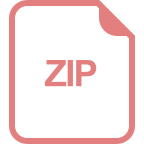
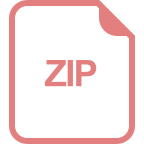
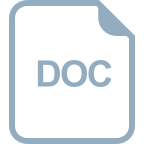
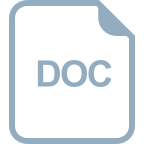
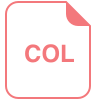
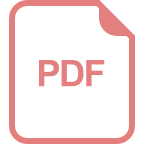
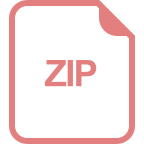