volatile在Linux多线程中的应用C语言例子
时间: 2023-10-02 09:13:28 浏览: 45
在 Linux 多线程编程中,`volatile` 一般用于多线程之间共享的变量,它可以保证数据的可见性和一致性,避免了因编译器优化而导致的数据不一致问题。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
volatile int counter = 0;
void *thread_func(void *arg) {
int i;
for (i = 0; i < 1000000; i++) {
counter++; // 对 counter 做自增操作
}
return NULL;
}
int main() {
pthread_t t1, t2;
pthread_create(&t1, NULL, thread_func, NULL);
pthread_create(&t2, NULL, thread_func, NULL);
pthread_join(t1, NULL);
pthread_join(t2, NULL);
printf("counter = %d\n", counter); // 输出 counter 的值
return 0;
}
```
在上面的例子中,我们定义了一个全局变量 `counter`,它被两个线程同时访问。在 `thread_func` 函数中,每个线程会对 `counter` 做 1000000 次自增操作。在主线程中,我们通过输出 `counter` 的值来验证两个线程是否都正确地修改了它。如果我们不使用 `volatile` 修饰 `counter`,则可能会出现多种问题,如线程之间的数据不一致等。但是使用 `volatile`,我们可以保证 `counter` 的值在所有线程中是一致的。
注意,`volatile` 不能保证原子性,如果需要保证原子性,需要使用其他的同步机制,如互斥锁、读写锁等。
相关推荐
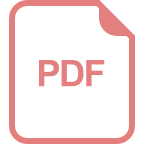
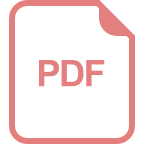














