c# 木工二维优化下料算法代码
时间: 2023-10-04 17:10:46 浏览: 107
以下是一个简单的 C# 二维优化下料算法的代码示例:
```csharp
using System;
using System.Collections.Generic;
namespace WoodCutting
{
class Program
{
static void Main(string[] args)
{
List<WoodPiece> woodPieces = new List<WoodPiece>();
woodPieces.Add(new WoodPiece(4, 8));
woodPieces.Add(new WoodPiece(5, 7));
woodPieces.Add(new WoodPiece(6, 6));
woodPieces.Add(new WoodPiece(8, 4));
woodPieces.Add(new WoodPiece(10, 3));
List<WoodCut> woodCuts = OptimizeWoodCutting(woodPieces, 10, 20);
Console.WriteLine("Optimized Wood Cuts:");
foreach (WoodCut cut in woodCuts)
{
Console.WriteLine("Wood Piece: {0}x{1}", cut.Width, cut.Height);
Console.WriteLine("Cut Location: ({0}, {1})", cut.X, cut.Y);
}
}
static List<WoodCut> OptimizeWoodCutting(List<WoodPiece> woodPieces, int boardWidth, int boardHeight)
{
List<WoodCut> woodCuts = new List<WoodCut>();
int x = 0;
int y = 0;
foreach (WoodPiece piece in woodPieces)
{
if (x + piece.Width <= boardWidth && y + piece.Height <= boardHeight)
{
woodCuts.Add(new WoodCut(piece.Width, piece.Height, x, y));
x += piece.Width;
}
else
{
x = 0;
y += piece.Height;
if (y > boardHeight)
{
throw new Exception("Board is not big enough to fit all wood pieces.");
}
woodCuts.Add(new WoodCut(piece.Width, piece.Height, x, y));
x += piece.Width;
}
}
return woodCuts;
}
}
class WoodPiece
{
public int Width { get; set; }
public int Height { get; set; }
public WoodPiece(int width, int height)
{
Width = width;
Height = height;
}
}
class WoodCut
{
public int Width { get; set; }
public int Height { get; set; }
public int X { get; set; }
public int Y { get; set; }
public WoodCut(int width, int height, int x, int y)
{
Width = width;
Height = height;
X = x;
Y = y;
}
}
}
```
在这个例子中,我们有一个 `WoodPiece` 类来表示木材,一个 `WoodCut` 类来表示切割的木材块,以及一个名为 `OptimizeWoodCutting` 的函数来执行切割操作。在这个函数中,我们将每个木材块与木板的尺寸进行比较,并将其放置在可用空间内。如果木材块的宽度大于木板的宽度,则将其放置在下一行的开头。
请注意,此代码示例仅适用于基本的二维优化下料算法。实际应用中,您可能需要考虑更多因素,例如木材的形状和大小、切割顺序以及更高级的算法。
阅读全文
相关推荐
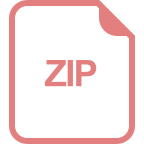
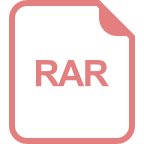
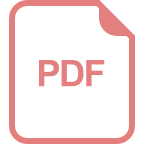
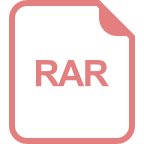
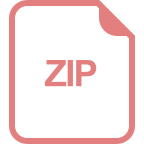
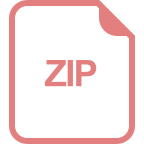
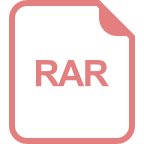
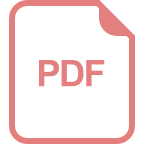
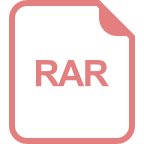
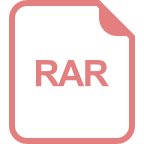
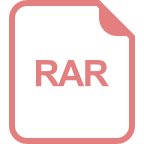
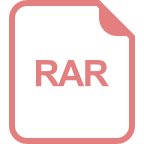
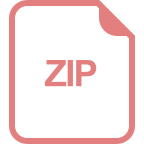