qt创建4个共享内存并存入图片,然后分别读取共享内存中的图片显示
时间: 2024-03-21 08:39:35 浏览: 237
好的,这个问题涉及到共享内存和图像的处理,需要用到Qt的相关功能。我来简单描述一下解决思路:
1. 创建4个共享内存对象,可以使用Qt的QSharedMemory类。
```c++
QSharedMemory sharedMemory1("Image1");
QSharedMemory sharedMemory2("Image2");
QSharedMemory sharedMemory3("Image3");
QSharedMemory sharedMemory4("Image4");
```
2. 加载图片到内存中,可以使用Qt的QPixmap类。
```c++
QPixmap image1("image1.jpg");
QPixmap image2("image2.jpg");
QPixmap image3("image3.jpg");
QPixmap image4("image4.jpg");
```
3. 将图片数据写入共享内存中。
```c++
sharedMemory1.create(image1.byteCount());
sharedMemory1.lock();
char *to = (char*)sharedMemory1.data();
const char *from = image1.constBits();
memcpy(to, from, qMin(sharedMemory1.size(), image1.byteCount()));
sharedMemory1.unlock();
sharedMemory2.create(image2.byteCount());
sharedMemory2.lock();
to = (char*)sharedMemory2.data();
from = image2.constBits();
memcpy(to, from, qMin(sharedMemory2.size(), image2.byteCount()));
sharedMemory2.unlock();
sharedMemory3.create(image3.byteCount());
sharedMemory3.lock();
to = (char*)sharedMemory3.data();
from = image3.constBits();
memcpy(to, from, qMin(sharedMemory3.size(), image3.byteCount()));
sharedMemory3.unlock();
sharedMemory4.create(image4.byteCount());
sharedMemory4.lock();
to = (char*)sharedMemory4.data();
from = image4.constBits();
memcpy(to, from, qMin(sharedMemory4.size(), image4.byteCount()));
sharedMemory4.unlock();
```
4. 读取共享内存中的图片数据并显示在界面上。
```c++
QPixmap pixmap1;
sharedMemory1.lock();
pixmap1.loadFromData((const uchar*)sharedMemory1.constData(), sharedMemory1.size());
sharedMemory1.unlock();
ui->label1->setPixmap(pixmap1);
QPixmap pixmap2;
sharedMemory2.lock();
pixmap2.loadFromData((const uchar*)sharedMemory2.constData(), sharedMemory2.size());
sharedMemory2.unlock();
ui->label2->setPixmap(pixmap2);
QPixmap pixmap3;
sharedMemory3.lock();
pixmap3.loadFromData((const uchar*)sharedMemory3.constData(), sharedMemory3.size());
sharedMemory3.unlock();
ui->label3->setPixmap(pixmap3);
QPixmap pixmap4;
sharedMemory4.lock();
pixmap4.loadFromData((const uchar*)sharedMemory4.constData(), sharedMemory4.size());
sharedMemory4.unlock();
ui->label4->setPixmap(pixmap4);
```
这样就可以通过共享内存实现多个进程之间的图像数据共享了。当然,还需要根据实际情况进行相关的错误处理和其他逻辑的完善。
阅读全文
相关推荐
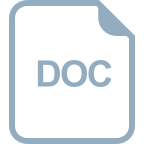
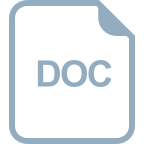
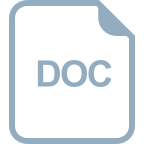


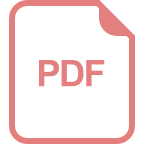
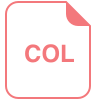
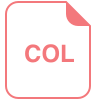

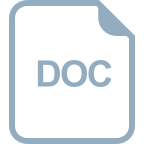
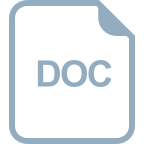
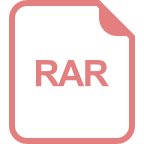
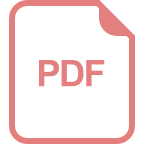
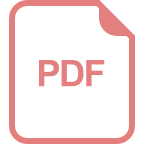
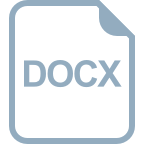
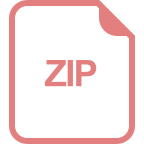
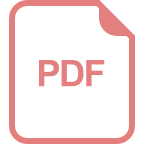
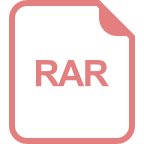