如何基于stable diffusion训练出自己的模型,给出详细的python代码
时间: 2024-05-04 13:22:26 浏览: 350
Stable Diffusion是一种用于生成模型训练的方法,可以用于训练自己的生成模型。下面是一个简单的例子,演示如何使用Python和PyTorch实现Stable Diffusion。
首先,需要安装以下依赖项:
- PyTorch
- NumPy
- scipy
- tqdm
安装完成后,可以使用以下代码实现Stable Diffusion:
```python
import torch
import numpy as np
from scipy import stats
from tqdm import tqdm
# 设定随机数生成器的种子
torch.manual_seed(0)
np.random.seed(0)
# 定义模型类
class MyModel(torch.nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.linear = torch.nn.Linear(1, 1)
def forward(self, x):
return self.linear(x)
# 定义模型超参数
model = MyModel()
num_steps = 1000
step_size = 0.1
noise_size = 0.1
# 定义数据
x_train = torch.tensor(np.linspace(-1, 1, 100)).unsqueeze(1).float()
y_train = x_train + torch.randn_like(x_train) * 0.1
# 定义优化器
optimizer = torch.optim.SGD(model.parameters(), lr=0.1)
# 定义稳定扰动采样函数
def stable_diffusion_sample(x, model, num_steps, step_size, noise_size):
for i in range(num_steps):
noise = torch.randn_like(x) * noise_size
x = x + step_size * model(x + noise) + noise
return x
# 训练模型
for i in tqdm(range(1000)):
idx = np.random.choice(len(x_train), size=16, replace=False)
x_batch = x_train[idx]
y_batch = y_train[idx]
optimizer.zero_grad()
y_pred = model(stable_diffusion_sample(x_batch, model, num_steps, step_size, noise_size))
loss = (y_pred - y_batch).abs().mean()
loss.backward()
optimizer.step()
if i % 100 == 0:
print(f"Loss: {loss.item()}")
# 测试模型
x_test = torch.tensor(np.linspace(-1, 1, 100)).unsqueeze(1).float()
y_test = x_test + torch.randn_like(x_test) * 0.1
y_pred = []
with torch.no_grad():
for x in x_test:
y_samples = [model(stable_diffusion_sample(x, model, num_steps, step_size, noise_size)) for _ in range(100)]
y_pred.append(torch.stack(y_samples).mean())
y_pred = torch.stack(y_pred)
# 计算测试集上的误差
test_loss = (y_pred - y_test).abs().mean()
print(f"Test Loss: {test_loss.item()}")
# 绘制结果
import matplotlib.pyplot as plt
plt.scatter(x_train, y_train, label='train')
plt.scatter(x_test, y_test, label='test')
plt.plot(x_test, y_pred, label='pred')
plt.legend()
plt.show()
```
在这个例子中,我们使用Stable Diffusion训练一个简单的线性回归模型。我们首先定义了一个包含一个线性层的模型类,然后使用PyTorch的优化器和稳定扰动采样函数来训练模型。在训练过程中,我们随机选择16个样本进行梯度下降,并在每个训练步骤中打印损失。最后,我们使用训练好的模型在测试集上进行预测,并计算测试集上的平均绝对误差。最后,我们将训练集、测试集和模型预测可视化。
阅读全文
相关推荐
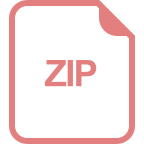
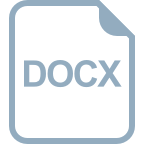
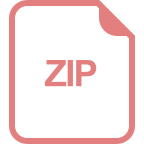

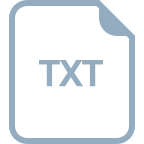
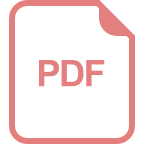
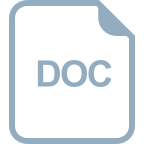
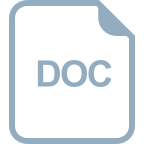





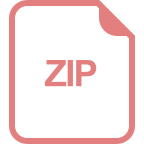
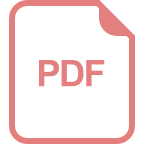
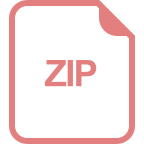