JSON的属性值是xml,如何提取xml C语言 举例
时间: 2024-03-05 10:52:40 浏览: 83
假设我们有一个JSON字符串,其中属性值是一个XML字符串,如下所示:
```json
{
"name": "John",
"age": 30,
"xml_data": "<person><name>Tom</name><age>25</age></person>"
}
```
我们可以使用第三方库cJSON来解析JSON数据,并使用libxml2库来解析XML数据。下面是一个简单的示例程序,可以提取xml_data中的name和age元素:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libxml/parser.h>
#include <libxml/tree.h>
#include "cJSON.h"
void parse_xml_data(const char *xml_data);
int main()
{
const char *json_data = "{\"name\":\"John\",\"age\":30,\"xml_data\":\"<person><name>Tom</name><age>25</age></person>\"}";
cJSON *root = cJSON_Parse(json_data);
if (root == NULL) {
printf("Failed to parse JSON data.\n");
return -1;
}
cJSON *xml_data = cJSON_GetObjectItem(root, "xml_data");
if (xml_data == NULL) {
printf("Failed to get xml_data.\n");
cJSON_Delete(root);
return -1;
}
parse_xml_data(xml_data->valuestring);
cJSON_Delete(root);
return 0;
}
void parse_xml_data(const char *xml_data)
{
xmlDocPtr doc = xmlReadMemory(xml_data, strlen(xml_data), NULL, NULL, 0);
if (doc == NULL) {
printf("Failed to parse XML data.\n");
return;
}
xmlNodePtr root = xmlDocGetRootElement(doc);
for (xmlNodePtr node = root->children; node != NULL; node = node->next) {
if (node->type == XML_ELEMENT_NODE) {
if (xmlStrcmp(node->name, (const xmlChar *)"name") == 0) {
printf("Name: %s\n", xmlNodeGetContent(node));
} else if (xmlStrcmp(node->name, (const xmlChar *)"age") == 0) {
printf("Age: %s\n", xmlNodeGetContent(node));
}
}
}
xmlFreeDoc(doc);
}
```
在这个示例程序中,我们首先使用cJSON库解析JSON数据,然后获取xml_data属性值,并将其传递给parse_xml_data函数。在parse_xml_data函数中,我们使用libxml2库解析XML数据,提取name和age元素的内容,并将其输出到控制台上。
阅读全文
相关推荐
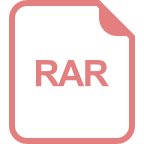
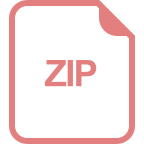
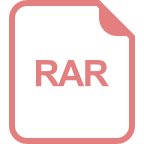
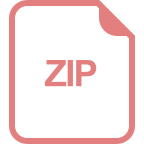
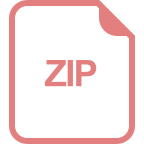
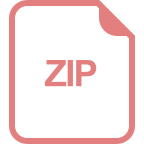
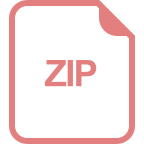
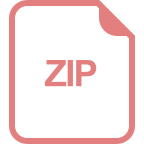
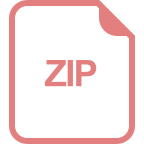
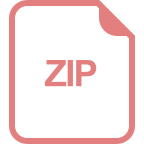
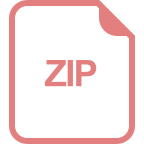
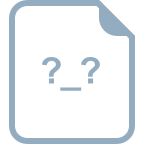
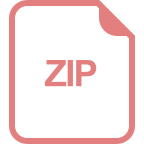
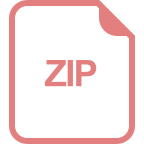
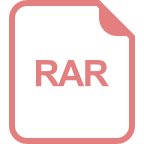