应用程序启动后,显示界面如图 1 所示。 图 1 程序主界面 2、创建一个后台服务,该服务启动后在一个新的线程中持续地以 1 秒间隔更新 通知栏,交替显示两个不同的图标,如图 2、图 3 所示
时间: 2024-01-16 12:04:10 浏览: 26
好的,您的问题是如何在 Android 应用程序中创建一个后台服务,并在该服务中更新通知栏,交替显示两个不同的图标。我可以给您提供一些思路和代码示例。
1. 创建一个后台服务
在 Android 中,我们可以通过创建一个 Service 来实现后台服务。Service 是一个 Android 组件,它可以在后台执行长时间运行的任务,而不需要与用户界面进行交互。
下面是一个创建后台服务的示例代码:
```java
public class MyService extends Service {
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// 在这里执行后台任务
return START_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
// 如果不需要绑定服务,则返回 null
return null;
}
}
```
在上面的代码中,我们创建了一个名为 MyService 的后台服务,并在 onStartCommand() 方法中执行后台任务。返回值 START_STICKY 表示如果系统在内存不足时杀死服务,则会尝试重新启动服务。
2. 更新通知栏,交替显示两个不同的图标
要更新通知栏,我们可以使用 NotificationManager 类。该类提供了一些方法来创建和更新通知。
下面是一个更新通知栏,交替显示两个不同的图标的示例代码:
```java
public class MyService extends Service {
private NotificationManager mNotificationManager;
private boolean mIsIcon1Shown = true;
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
mNotificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
// 创建两个不同的图标
int icon1 = R.drawable.icon1;
int icon2 = R.drawable.icon2;
// 创建通知
Notification notification = new Notification.Builder(this)
.setContentTitle("My Notification")
.setContentText("Hello, world!")
.setSmallIcon(mIsIcon1Shown ? icon1 : icon2)
.build();
// 更新通知栏
mNotificationManager.notify(0, notification);
// 交替显示两个不同的图标
mIsIcon1Shown = !mIsIcon1Shown;
// 每隔 1 秒更新一次通知栏
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
onStartCommand(null, 0, 0);
}
}, 1000);
return START_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
```
在上面的代码中,我们在 onStartCommand() 方法中创建了一个 Notification 对象,并设置了通知的标题、内容和小图标。然后,我们调用 NotificationManager.notify() 方法来更新通知栏。
为了交替显示两个不同的图标,我们使用了一个布尔值 mIsIcon1Shown 来表示当前是否显示第一个图标。在每次更新通知栏时,我们根据 mIsIcon1Shown 的值来设置小图标,并将其取反,以便下一次更新时显示另一个图标。
最后,我们使用 Handler.postDelayed() 方法来定时执行后台任务,并在每次执行后台任务时调用 onStartCommand() 方法来更新通知栏。
相关推荐
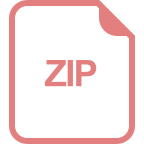
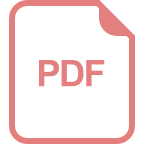
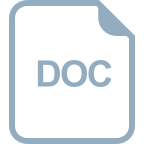














